帮我用python 写一个贪吃蛇
时间: 2023-07-01 17:11:19 浏览: 37
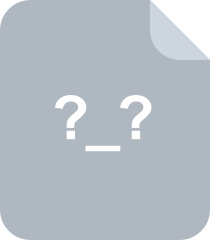
用python写一个贪吃蛇小游戏
好的,以下是一个简单的贪吃蛇游戏的Python代码:
```python
import pygame
import random
# 初始化窗口
pygame.init()
WIDTH, HEIGHT = 640, 480
screen = pygame.display.set_mode((WIDTH, HEIGHT))
pygame.display.set_caption("贪吃蛇")
# 定义颜色
BLACK = (0, 0, 0)
WHITE = (255, 255, 255)
RED = (255, 0, 0)
GREEN = (0, 255, 0)
BLUE = (0, 0, 255)
# 定义贪吃蛇类
class Snake:
def __init__(self):
self.body = [(WIDTH//2, HEIGHT//2)]
self.direction = (0, -1)
def move(self):
x, y = self.body[0]
dx, dy = self.direction
self.body.insert(0, (x+dx, y+dy))
self.body.pop()
def grow(self):
x, y = self.body[0]
dx, dy = self.direction
self.body.insert(0, (x+dx, y+dy))
def change_direction(self, direction):
self.direction = direction
# 定义食物类
class Food:
def __init__(self):
self.pos = (random.randint(0, WIDTH), random.randint(0, HEIGHT))
def respawn(self):
self.pos = (random.randint(0, WIDTH), random.randint(0, HEIGHT))
# 创建贪吃蛇和食物
snake = Snake()
food = Food()
# 游戏循环
clock = pygame.time.Clock()
score = 0
running = True
while running:
# 处理事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
elif event.type == pygame.KEYDOWN:
if event.key == pygame.K_UP:
snake.change_direction((0, -1))
elif event.key == pygame.K_DOWN:
snake.change_direction((0, 1))
elif event.key == pygame.K_LEFT:
snake.change_direction((-1, 0))
elif event.key == pygame.K_RIGHT:
snake.change_direction((1, 0))
# 移动贪吃蛇
snake.move()
# 判断是否吃到食物
if snake.body[0] == food.pos:
food.respawn()
snake.grow()
score += 10
# 判断是否撞墙或撞到自己
if snake.body[0][0] < 0 or snake.body[0][0] >= WIDTH or snake.body[0][1] < 0 or snake.body[0][1] >= HEIGHT:
running = False
for i in range(1, len(snake.body)):
if snake.body[0] == snake.body[i]:
running = False
# 绘制画面
screen.fill(WHITE)
for x, y in snake.body:
pygame.draw.rect(screen, GREEN, (x, y, 10, 10))
pygame.draw.rect(screen, RED, (food.pos[0], food.pos[1], 10, 10))
pygame.draw.rect(screen, BLACK, (0, 0, WIDTH, HEIGHT), 1)
font = pygame.font.SysFont("Arial", 20)
text = font.render("Score: {}".format(score), True, BLUE)
screen.blit(text, (10, 10))
pygame.display.update()
# 控制帧率
clock.tick(10)
# 退出游戏
pygame.quit()
```
这个代码使用了Pygame库来创建游戏窗口和处理事件,实现了基本的贪吃蛇游戏逻辑。你可以自己试着运行一下这个代码,并在此基础上进行修改和优化。
阅读全文
相关推荐

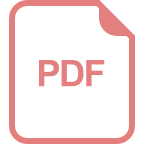
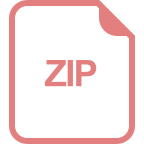
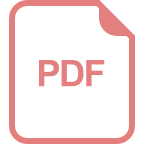
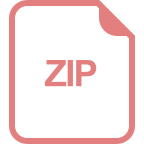
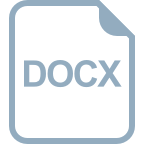
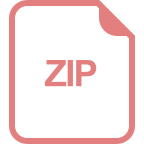