c++编写一个完整可运行的词法分析器
时间: 2024-05-03 15:17:41 浏览: 12
好的,下面是一个简单的C++词法分析器示例,可以读取一个文本文件并输出其所有的单词。
```cpp
#include <iostream>
#include <fstream>
#include <string>
#include <vector>
#include <cstdlib>
using namespace std;
// 定义 Token 结构体,存储单词和其类型
struct Token {
string lexeme; // 单词
string type; // 类型
};
// 判断字符是否为字母或下划线
bool isLetter(char c) {
return (c >= 'a' && c <= 'z') || (c >= 'A' && c <= 'Z') || c == '_';
}
// 判断字符是否为数字
bool isDigit(char c) {
return c >= '0' && c <= '9';
}
// 判断是否为关键字
bool isKeyword(string s) {
vector<string> keywords = {"if", "else", "while", "for", "int", "float", "double"};
for (auto keyword : keywords) {
if (s == keyword) {
return true;
}
}
return false;
}
// 词法分析函数
vector<Token> lexer(string input) {
vector<Token> tokens;
int i = 0;
while (i < input.length()) {
// 跳过空格和换行符
if (input[i] == ' ' || input[i] == '\n') {
i++;
continue;
}
// 判断是否为关键字或标识符
if (isLetter(input[i])) {
int j = i;
while (j < input.length() && (isLetter(input[j]) || isDigit(input[j]))) {
j++;
}
string lexeme = input.substr(i, j - i);
string type = isKeyword(lexeme) ? "keyword" : "identifier";
tokens.push_back({lexeme, type});
i = j;
}
// 判断是否为数字
else if (isDigit(input[i])) {
int j = i;
while (j < input.length() && isDigit(input[j])) {
j++;
}
string lexeme = input.substr(i, j - i);
tokens.push_back({lexeme, "number"});
i = j;
}
// 判断是否为符号
else {
string lexeme = input.substr(i, 1);
if (lexeme == "+" || lexeme == "-" || lexeme == "*" || lexeme == "/") {
tokens.push_back({lexeme, "operator"});
} else if (lexeme == "=") {
tokens.push_back({lexeme, "assign"});
} else if (lexeme == "(" || lexeme == ")") {
tokens.push_back({lexeme, "paren"});
}
i++;
}
}
return tokens;
}
int main(int argc, char const *argv[]) {
// 读取文件内容
if (argc < 2) {
cerr << "Usage: " << argv[0] << " FILENAME" << endl;
return 1;
}
string filename = argv[1];
ifstream ifs(filename);
if (!ifs) {
cerr << "Failed to open file: " << filename << endl;
return 1;
}
string input((istreambuf_iterator<char>(ifs)), (istreambuf_iterator<char>()));
// 进行词法分析
vector<Token> tokens = lexer(input);
// 输出结果
for (auto token : tokens) {
cout << token.lexeme << "\t" << token.type << endl;
}
return 0;
}
```
该程序通过定义一个 Token 结构体来存储每个单词及其类型,然后使用一个 lexer 函数对文本进行词法分析并生成 Token 列表。在主函数中,读取文件内容并调用 lexer 函数,最后输出 Token 列表中的每个元素。
注意:该示例代码只是一个简单的演示,实际编写的词法分析器需要考虑更多细节和错误处理。
相关推荐
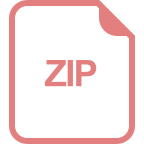
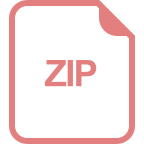
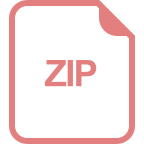
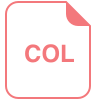
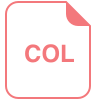
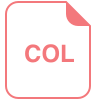
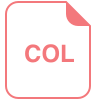










