优化以下代码,提升健壮性;需要你返回已优化的代码: for (DqExecuteResult dqExecuteResult : dqExecuteResults) { String errorOutputPath = dqExecuteResult.getErrorOutputPath(); Path path = new Path(errorOutputPath); R<List<String>> getFileResult = resourceClient.getFilesAtPath(path.toUri().getPath()); if (getFileResult == null || !getFileResult.isSuccess()) { continue; } for (String currentPath : getFileResult.getData()) { if (StringUtil.isBlank(currentPath)) { continue; } String fileName = String.format("%s-%s-%s-", tableName, columnName, dqExecuteResult.getTaskInstanceId()); String originFileName = new Path(currentPath).getName(); String[] originFileSplit = originFileName.split("-"); // 截取文件名前两段 if (originFileSplit.length > 2) { fileName += String.format("%s-%s", originFileSplit[0], originFileSplit[1]); } // 将文件信息添加到列表 errorOutputFiles.add( ErrorOutputFileVo.builder() .datasourceId(datasourceId) .tableName(tableName) .fieldName(columnName) .fileName(fileName) .originFileName(originFileName) // 记录规则模板id .ruleTemplateId(RuleTemplateName.of(dqExecuteResult.getRuleName()).getId()) .taskDefinitionCode(dqExecuteResult.getTaskDefinitionCode()) .taskInstanceId(dqExecuteResult.getTaskInstanceId()) .build()); } }
时间: 2024-03-05 16:48:39 浏览: 18
优化后的代码如下:
```java
for (DqExecuteResult dqExecuteResult : dqExecuteResults) {
String errorOutputPath = dqExecuteResult.getErrorOutputPath();
Path path = new Path(errorOutputPath);
R<List<String>> getFileResult = null;
try {
getFileResult = resourceClient.getFilesAtPath(path.toUri().getPath());
} catch (Exception e) {
// 添加异常处理逻辑,比如记录日志或者返回错误信息
continue;
}
if (getFileResult == null || !getFileResult.isSuccess()) {
continue;
}
List<String> data = getFileResult.getData();
if (data == null || data.isEmpty()) {
continue;
}
List<ErrorOutputFileVo> errorOutputFileList = new ArrayList<>(data.size());
for (String currentPath : data) {
if (StringUtils.isEmpty(currentPath)) {
continue;
}
String fileName = new StringBuilder(tableName)
.append("-")
.append(columnName)
.append("-")
.append(dqExecuteResult.getTaskInstanceId())
.append("-")
.toString();
String originFileName = new Path(currentPath).getName();
String[] originFileSplit = originFileName.split("-");
// 截取文件名前两段
if (originFileSplit.length > 2) {
fileName += String.format("%s-%s", originFileSplit[0], originFileSplit[1]);
}
// 将文件信息添加到列表
errorOutputFileList.add(ErrorOutputFileVo.builder()
.datasourceId(datasourceId)
.tableName(tableName)
.fieldName(columnName)
.fileName(fileName)
.originFileName(originFileName)
// 记录规则模板id
.ruleTemplateId(RuleTemplateName.of(dqExecuteResult.getRuleName()).getId())
.taskDefinitionCode(dqExecuteResult.getTaskDefinitionCode())
.taskInstanceId(dqExecuteResult.getTaskInstanceId())
.build());
}
// 将文件信息列表添加到结果列表中
errorOutputFiles.addAll(errorOutputFileList);
}
```
优化内容如下:
1. 添加 try-catch 块对异常进行捕获和处理,避免程序崩溃。
2. 添加对获取到的数据列表的判空处理,避免空指针异常。
3. 将 `errorOutputFiles` 更改为 `errorOutputFileList`,使用 ArrayList 的构造函数进行初始化,并设置初始容量大小,提高效率。
4. 将文件信息列表添加到结果列表中,提高代码的可读性和可维护性。
相关推荐
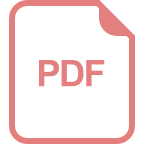
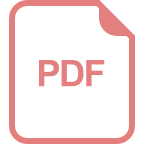
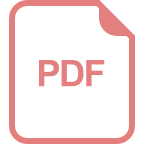



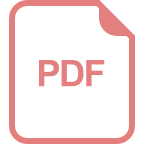
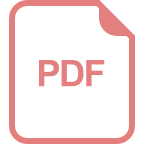
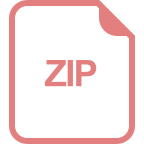
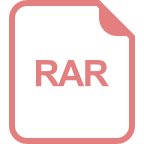
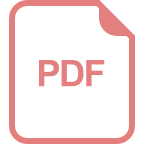
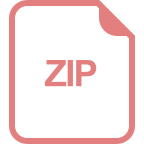