用python画图案
时间: 2024-10-14 19:07:55 浏览: 5
在Python中,我们可以使用各种库如turtle、PIL(Python Imaging Library)或者matplotlib等来绘制图形和图案。其中,turtle模块特别适合初学者,因为它提供了一个类似Turtle图形绘图语言的接口,可以轻松创建各种形状。
以下是一个简单的例子,展示如何使用turtle模块绘制一个正方形:
```python
import turtle
# 创建一个turtle对象
t = turtle.Turtle()
# 设置线条颜色和宽度
t.pencolor("blue")
t.pensize(5)
# 开始绘画
for _ in range(4):
t.forward(100) # 向前移动100像素
t.right(90) # 右转90度
# 结束绘制并关闭窗口
t.hideturtle()
turtle.done()
```
如果你想绘制更复杂的图案,比如笑脸、心形或者其他自定义形状,你可以通过控制turtle的位置、方向以及改变颜色和线条样式等来实现。如果需要制作更专业的图像,可以考虑使用PIL或matplotlib进行更高级的图像处理和可视化。
相关问题
python画花瓣图案
Python可以使用各种库来画花瓣图案,其中比较常用的是turtle库和matplotlib库。下面我将介绍使用这两个库来画花瓣图案的方法。
使用turtle库画花瓣图案的步骤如下:
1. 导入turtle库:`import turtle`
2. 创建一个画布:`turtle.Screen()`
3. 创建一个画笔:`pen = turtle.Turtle()`
4. 设置画笔的颜色、形状等属性:`pen.color("red")`
5. 使用循环语句画出花瓣图案,可以使用for循环或while循环,具体的花瓣形状可以根据需求自行设计。
6. 结束绘制:`turtle.done()`
使用matplotlib库画花瓣图案的步骤如下:
1. 导入matplotlib库:`import matplotlib.pyplot as plt`
2. 创建一个画布:`fig, ax = plt.subplots()`
3. 设置画布的大小和背景颜色等属性:`fig.set_size_inches(6, 6)`
4. 使用matplotlib提供的函数绘制花瓣图案,可以使用plot函数、scatter函数等,具体的花瓣形状可以根据需求自行设计。
5. 显示图像:`plt.show()`
python画青花瓷图案
把文字打印成"青花蓝"并且使用Python画出青花瓷图案可以通过使用Python的绘图库来实现。可以使用Pillow库来创建一个画布,然后通过画图函数和循环来绘制出青花瓷的花纹。
以下是一个使用Python绘制青花瓷图案的示例代码:
```python
from PIL import Image, ImageDraw
# 创建画布和绘图对象
canvas_width = 500
canvas_height = 500
canvas = Image.new('RGB', (canvas_width, canvas_height), 'white')
draw = ImageDraw.Draw(canvas)
# 绘制青花瓷花纹
pattern_color = (0, 0, 128) # 青花蓝颜色
pattern_size = 50 # 花纹的大小
for x in range(0, canvas_width, pattern_size):
for y in range(0, canvas_height, pattern_size):
# 绘制花纹
draw.rectangle((x, y, x + pattern_size, y + pattern_size), fill=pattern_color)
# 保存绘图结果
canvas.save('青花瓷图案.png')
# 显示绘图结果
canvas.show()
```
这段代码将在一个500x500像素的画布上绘制青花瓷的图案,并将结果保存为"青花瓷图案.png"文件。你可以根据需要调整画布大小、花纹颜色和大小。
相关推荐
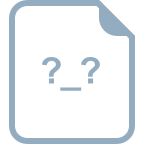
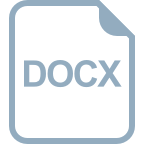













