帮我实现一个C++的图书管理系统
时间: 2024-03-04 13:49:45 浏览: 66
好的,下面是一个简单的图书管理系统的C++代码示例:
```cpp
#include <iostream>
#include <vector>
#include <string>
using namespace std;
class Book {
private:
string title;
string author;
string publisher;
int publicationYear;
int numPages;
public:
Book(string title, string author, string publisher, int publicationYear, int numPages) {
this->title = title;
this->author = author;
this->publisher = publisher;
this->publicationYear = publicationYear;
this->numPages = numPages;
}
string getTitle() {
return title;
}
string getAuthor() {
return author;
}
string getPublisher() {
return publisher;
}
int getPublicationYear() {
return publicationYear;
}
int getNumPages() {
return numPages;
}
};
class Library {
private:
vector<Book> books;
public:
void addBook(Book book) {
books.push_back(book);
}
void removeBook(string title) {
for (int i = 0; i < books.size(); i++) {
if (books[i].getTitle() == title) {
books.erase(books.begin() + i);
break;
}
}
}
void printBooks() {
for (int i = 0; i < books.size(); i++) {
cout << "Title: " << books[i].getTitle() << endl;
cout << "Author: " << books[i].getAuthor() << endl;
cout << "Publisher: " << books[i].getPublisher() << endl;
cout << "Publication Year: " << books[i].getPublicationYear() << endl;
cout << "Number of Pages: " << books[i].getNumPages() << endl << endl;
}
}
};
int main() {
Library library;
Book book1("The Catcher in the Rye", "J.D. Salinger", "Little, Brown and Company", 1951, 277);
Book book2("1984", "George Orwell", "Secker & Warburg", 1949, 328);
Book book3("To Kill a Mockingbird", "Harper Lee", "J.B. Lippincott & Co.", 1960, 281);
library.addBook(book1);
library.addBook(book2);
library.addBook(book3);
library.printBooks();
library.removeBook("1984");
library.printBooks();
return 0;
}
```
这个图书管理系统有两个类:`Book`和`Library`。`Book`类表示一本书,包含书名、作者、出版社、出版年份和页数等信息,`Library`类表示图书馆,包含一个`Book`对象的向量,可以添加、删除和打印书籍信息。在`main`函数中,我们使用`Library`类来管理三本书,并打印出它们的信息。然后,我们删除了一本书并再次打印了所有的书籍信息。
阅读全文
相关推荐





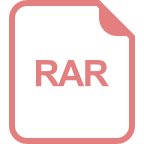



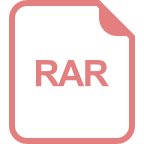





