用matlab编制一个程序,读取位图并显示在屏幕上,将图像数据化并显示结果,学会如何返回指定点的像素坐标。
时间: 2024-09-23 11:10:40 浏览: 15
在MATLAB中,你可以使用imread函数来读取位图文件,并使用imshow函数将其显示在屏幕上。以下是编写这样一个程序的基本步骤:
```matlab
% 1. 读取位图文件
img = imread('your_image_file.bmp'); % 将 'your_image_file.bmp' 替换为你要处理的实际图片路径
% 2. 显示图像
imshow(img);
title('Original Image');
% 3. 图像数据化
% MATLAB中的灰度图像数据是一个矩阵,索引对应于像素位置。你可以获取某个特定像素点的RGB值,例如(50, 70)位置:
% 如果是彩色图像,使用 img(50, 70, :) 获取该位置的颜色信息,如果是灰度图像则简化为 img(50, 70)
specific_pixel_color = img(50, 70, :);
% 显示特定像素的颜色
disp(['Pixel at (50, 70): ', mat2str(specific_pixel_color)]);
% 或者如果你想获取灰度值,如果是灰度图像,可以简单地这样做:
gray_value = img(50, 70);
disp(['Gray value at (50, 70): ', num2str(gray_value)]);
% 4. 返回指定点的像素坐标
function [row, col] = getPixelCoord(image, pixel_val)
% 搜索图像中给定颜色的像素坐标
[row, col] = find(image == pixel_val);
end
[row, col] = getPixelCoord(img, specific_pixel_color); % 示例调用
if ~isempty(row)
disp(['Pixel with color found at (', num2str(row), ', ', num2str(col), ')']);
else
disp('Color not found in the image.');
end
```
记得替换`'your_image_file.bmp'`为你实际需要读取的位图文件路径。这个程序演示了基本的位图操作,包括显示、获取像素值以及搜索特定颜色。
相关推荐
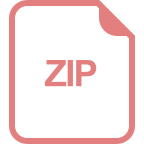
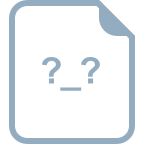
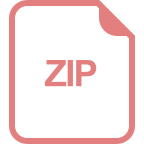
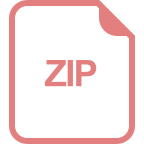
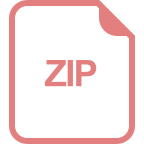
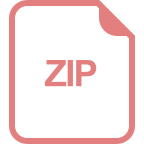
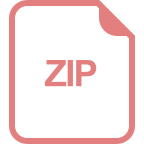
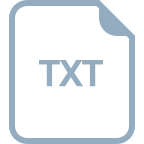
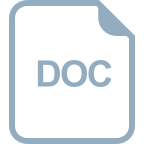
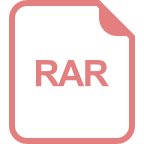
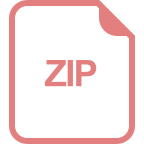
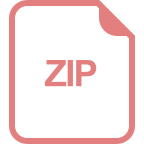
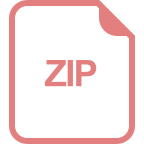
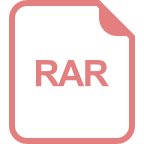
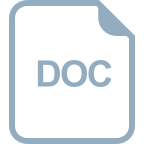