用java和MySQL写一个银行开户功能
时间: 2024-04-02 22:36:49 浏览: 18
好的,以下是一个简单的Java程序,用于将用户的开户信息存储到MySQL数据库中:
首先,你需要用Java连接到MySQL数据库,可以用JDBC驱动来实现。
```java
import java.sql.*;
public class BankAccountCreation {
static final String JDBC_DRIVER = "com.mysql.jdbc.Driver";
static final String DB_URL = "jdbc:mysql://localhost/Bank";
static final String USER = "username";
static final String PASS = "password";
public static void main(String[] args) {
Connection conn = null;
Statement stmt = null;
try{
Class.forName(JDBC_DRIVER);
conn = DriverManager.getConnection(DB_URL,USER,PASS);
stmt = conn.createStatement();
String sql = "INSERT INTO BankAccounts (Name, AccountNumber, Balance) " +
"VALUES ('John Doe', '1234567890', 1000)";
stmt.executeUpdate(sql);
System.out.println("Bank account created successfully!");
stmt.close();
conn.close();
}catch(SQLException se){
se.printStackTrace();
}catch(Exception e){
e.printStackTrace();
}finally{
try{
if(stmt!=null)
stmt.close();
}catch(SQLException se2){
}
try{
if(conn!=null)
conn.close();
}catch(SQLException se){
se.printStackTrace();
}
}
}
}
```
在上述代码中,我们将用户的姓名、账号和余额插入到一个名为“BankAccounts”的表中。
当你运行这个程序时,它将连接到MySQL数据库,创建一个名为“BankAccounts”的表,并将用户信息插入到该表中。
当然,你也可以将用户输入的数据作为变量传递给SQL语句中,以便根据用户输入的信息创建银行账户。
相关推荐
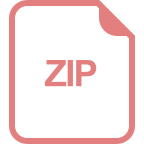
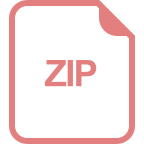














