WPF 通过路由实现两个控件的联动
时间: 2024-03-16 21:45:28 浏览: 18
WPF 中可以通过路由实现控件的联动,比如当一个控件的值改变时,另一个控件的值也跟着改变。
具体实现可以通过以下步骤:
1. 使用 `DependencyProperty` 定义一个依赖属性,用于存储控件的值。
2. 在控件的 XAML 中,将该依赖属性绑定到控件的值属性上。
3. 使用 `PropertyChangedCallback` 方法监听值的变化,在方法中通过路由查找找到需要联动的控件,并将其值更新。
以下是一个实现两个 `TextBox` 联动的示例代码:
```
public class TextBoxHelper
{
public static readonly DependencyProperty TextProperty =
DependencyProperty.RegisterAttached("Text", typeof(string), typeof(TextBoxHelper), new PropertyMetadata(null, OnTextChanged));
public static string GetText(DependencyObject obj)
{
return (string)obj.GetValue(TextProperty);
}
public static void SetText(DependencyObject obj, string value)
{
obj.SetValue(TextProperty, value);
}
private static void OnTextChanged(DependencyObject d, DependencyPropertyChangedEventArgs e)
{
// 获取路由根节点
var root = (FrameworkElement)Application.Current.MainWindow;
// 查找需要联动的控件
var textBoxes = FindVisualChildren<TextBox>(root);
// 更新控件的值
foreach (var textBox in textBoxes)
{
if (textBox != d)
{
textBox.Text = e.NewValue as string;
}
}
}
private static IEnumerable<T> FindVisualChildren<T>(DependencyObject obj) where T : DependencyObject
{
if (obj != null)
{
for (int i = 0; i < VisualTreeHelper.GetChildrenCount(obj); i++)
{
DependencyObject child = VisualTreeHelper.GetChild(obj, i);
if (child is T t)
{
yield return t;
}
foreach (T childOfChild in FindVisualChildren<T>(child))
{
yield return childOfChild;
}
}
}
}
}
```
在 XAML 中,将 `Text` 属性绑定到 `TextBoxHelper` 中定义的依赖属性,实现联动:
```
<TextBox local:TextBoxHelper.Text="{Binding Text1, Mode=TwoWay}" />
<TextBox local:TextBoxHelper.Text="{Binding Text2, Mode=TwoWay}" />
```
其中,`Text1` 和 `Text2` 分别为两个 `TextBox` 的值。
这样,当一个 `TextBox` 的值改变时,另一个 `TextBox` 的值也会跟着改变。
相关推荐
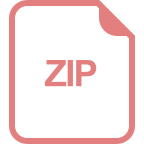
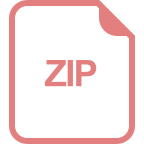
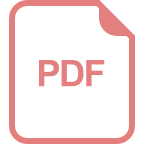














