java后端获取前端图片
时间: 2023-06-16 12:03:50 浏览: 80
Java后端获取前端图片可以通过以下步骤实现:
1. 前端将图片转换为Base64编码,并通过POST请求发送给后端。
2. 后端接收到请求后,读取POST请求中的Base64编码字符串。
3. 将Base64编码字符串转换为字节数组。
4. 将字节数组转换为图片格式,保存到本地或者存储到数据库中。
以下是一个简单的示例代码:
前端代码:
```html
<input type="file" accept="image/*" onchange="readImage(this)">
<script>
function readImage(input) {
if (input.files && input.files[0]) {
var reader = new FileReader();
reader.onload = function (e) {
var image = e.target.result;
//将图片转换为Base64编码,发送到后端
$.ajax({
type: "POST",
url: "/uploadImage",
data: { "image": image },
success: function (data) {
alert("上传成功");
},
error: function (xhr, textStatus, error) {
alert("上传失败:" + error);
}
});
};
reader.readAsDataURL(input.files[0]);
}
}
</script>
```
后端代码:
```java
@PostMapping("/uploadImage")
public String uploadImage(@RequestParam("image") String imageBase64) {
//将Base64编码字符串转换为字节数组
byte[] imageBytes = Base64.getDecoder().decode(imageBase64.split(",")[1]);
//将字节数组转换为图片格式
BufferedImage image = null;
try {
image = ImageIO.read(new ByteArrayInputStream(imageBytes));
} catch (IOException e) {
e.printStackTrace();
}
//保存图片到本地或者存储到数据库中
//...
return "success";
}
```
需要注意的是,在实际应用中,需要对上传的图片进行大小、格式等限制,以确保系统的安全性和稳定性。
相关推荐
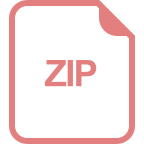
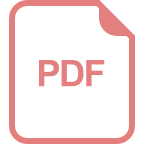
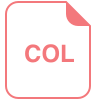
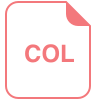
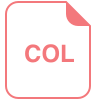











