Qt modem添加串口的自动检测
时间: 2023-09-25 22:11:10 浏览: 143
要实现Qt程序中串口的自动检测,可以使用QSerialPortInfo类来获取当前系统中所有可用的串口信息,并通过信号槽机制来实现串口的动态添加和移除。
具体实现步骤如下:
1. 在Qt项目中添加头文件#include<QSerialPortInfo>。
2. 在需要使用串口的类中定义一个QSerialPortInfo对象,并连接一个定时器信号槽。
3. 在定时器的槽函数中,使用QSerialPortInfo::availablePorts()函数获取当前系统中所有可用的串口信息。然后通过遍历可用串口的方式,判断系统中是否新增了串口或移除了串口。如果新增了串口,则可以将其添加到程序中;如果移除了串口,则可以将其从程序中移除。
下面是一个简单的示例代码:
```c++
#include <QSerialPortInfo>
#include <QTimer>
class SerialPortManager : public QObject
{
Q_OBJECT
public:
SerialPortManager(QObject *parent = 0);
~SerialPortManager();
public slots:
void checkSerialPort();
private:
QList<QSerialPortInfo> m_currentPorts;
QTimer *m_timer;
};
SerialPortManager::SerialPortManager(QObject *parent)
: QObject(parent)
{
m_timer = new QTimer(this);
connect(m_timer, SIGNAL(timeout()), this, SLOT(checkSerialPort()));
m_timer->start(1000); // 每秒检测一次串口
}
SerialPortManager::~SerialPortManager()
{
m_timer->stop();
delete m_timer;
}
void SerialPortManager::checkSerialPort()
{
QList<QSerialPortInfo> ports = QSerialPortInfo::availablePorts();
if (ports.count() == m_currentPorts.count()) {
// 串口数量没有变化,不需要处理
return;
}
// 遍历所有可用串口
foreach (QSerialPortInfo port, ports) {
if (!m_currentPorts.contains(port)) {
// 新增了一个串口
qDebug() << "Add serial port:" << port.portName();
// TODO: 在程序中添加该串口
m_currentPorts.append(port);
}
}
// 遍历当前已有串口
foreach (QSerialPortInfo port, m_currentPorts) {
if (!ports.contains(port)) {
// 移除了一个串口
qDebug() << "Remove serial port:" << port.portName();
// TODO: 在程序中移除该串口
m_currentPorts.removeOne(port);
}
}
}
```
在上述代码中,checkSerialPort()函数会每秒检测一次系统中可用的串口,并根据串口数量的变化来判断是否新增或移除了串口。如果新增了串口,则会输出该串口的名称,并将其添加到m_currentPorts列表中;如果移除了串口,则会输出该串口的名称,并将其从m_currentPorts列表中移除。程序中的TODO注释部分需要根据实际情况来实现,可以调用相应的函数来添加或移除串口。
阅读全文
相关推荐
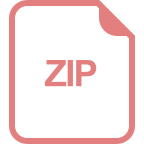
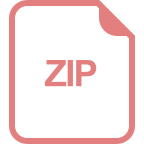
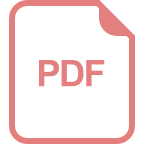















