代码利用多线程爬取豆瓣网站下标签为人文的书籍
时间: 2024-01-17 10:04:27 浏览: 24
好的,以下是一个使用 Python 多线程爬取豆瓣网站下标签为人文的书籍的示例代码:
```python
import requests
from bs4 import BeautifulSoup
from concurrent.futures import ThreadPoolExecutor
def scrape_book_info(url):
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
book_title = soup.find('div', {'class': 'title_wrapper'}).h1.text.strip()
book_author = soup.find('div', {'class': 'title_wrapper'}).h2.text.strip()
book_publisher = soup.find('div', {'id': 'info'}).find('span', text='出版社:').next_sibling.strip()
book_pubdate = soup.find('div', {'id': 'info'}).find('span', text='出版年:').next_sibling.strip()
book_rating = soup.find('strong', {'class': 'rating_num'}).text.strip()
print(f'{book_title} - {book_author} - {book_publisher} - {book_pubdate} - {book_rating}')
def scrape_books(tag, page):
url = f'https://book.douban.com/tag/{tag}?start={page*20}&type=T'
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
book_list = soup.find_all('li', {'class': 'subject-item'})
book_urls = [book.find('div', {'class': 'info'}).h2.a['href'] for book in book_list]
with ThreadPoolExecutor(max_workers=10) as executor:
executor.map(scrape_book_info, book_urls)
if __name__ == '__main__':
tag = '人文'
page = 0
while True:
scrape_books(tag, page)
page += 1
```
这段代码使用 requests 库和 BeautifulSoup 库进行网页爬取和信息提取。首先,定义了一个 `scrape_book_info` 函数用于解析每本书的信息,然后定义了一个 `scrape_books` 函数用于解析每个页面上的所有书籍信息。最后,在主函数中使用一个 while 循环不断爬取不同页面的书籍信息,直到所有页面都被爬取完毕。使用 `ThreadPoolExecutor` 类实现了多线程并发爬取,从而提高了爬取速度。
需要注意的是,这是一个简单示例,仅供参考。在实际使用中,需要考虑更多的因素,如反爬机制、异常处理、存储数据等。同时,也需要注意遵守网站的规定,避免对网站造成过大的负担。
相关推荐
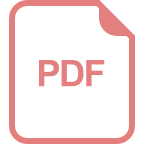
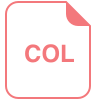
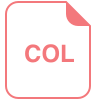
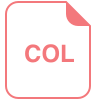
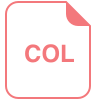
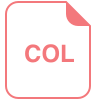









