android restfull post
时间: 2023-07-11 14:39:02 浏览: 107
下面是使用Java和Android Studio构建RESTful API POST请求的简单示例:
1. 添加依赖项
在项目的build.gradle文件中添加以下依赖项:
```
dependencies {
implementation 'com.android.volley:volley:1.1.1'
}
```
2. 创建POST请求
在你的Activity或Fragment中创建一个POST请求:
```
String url = "https://jsonplaceholder.typicode.com/posts";
// Post parameters
JSONObject postData = new JSONObject();
try {
postData.put("title", "My Title");
postData.put("body", "My Body");
postData.put("userId", 1);
} catch (JSONException e) {
e.printStackTrace();
}
// Request a string response from the provided URL.
JsonObjectRequest jsonObjectRequest = new JsonObjectRequest
(Request.Method.POST, url, postData, new Response.Listener<JSONObject>() {
@Override
public void onResponse(JSONObject response) {
Log.d("API Response", response.toString());
}
}, new Response.ErrorListener() {
@Override
public void onErrorResponse(VolleyError error) {
Log.e("API Error", error.toString());
}
});
// Add the request to the RequestQueue.
RequestQueue queue = Volley.newRequestQueue(this);
queue.add(jsonObjectRequest);
```
在上面的代码中,我们将一个JSONObject作为POST数据传递。你可以根据自己的需求定制此对象。
3. 解析响应
在监听器中,你可以解析API响应。例如,在上面的代码中,我们可以将响应转换为JSON对象:
```
JSONObject jsonObject = response.getJSONObject();
```
然后,你可以使用此对象中的数据执行其他操作。
这是一个简单的例子,但你可以根据自己的需求定制它。记得在你的AndroidManifest.xml文件中添加Internet权限。
阅读全文
相关推荐
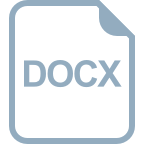
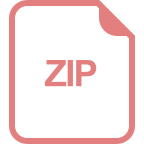
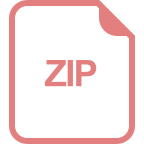















