题目:汽车销售信息管理系统 系统功能基本要求: 轿车信息,包括汽车的编号、型号、颜色、生产厂家、出厂日期、价格等;员工信息,包括员工编号、姓名、性别、年龄、藉贯、学历等; 客户信息,包括客户名称、联系方式、地址、业务联系记录等; 汽车销售信息,包括销售日期、汽车类型、颜色、数量、经手人等。按条件查询,并将销售报表打印输出。 用c++程序编写
时间: 2023-09-29 17:10:01 浏览: 31
好的,下面是一个简单的C++程序,用于实现上述汽车销售信息管理系统的基本功能要求:
```c++
#include <iostream>
#include <string>
#include <vector>
#include <algorithm>
using namespace std;
// 定义Car类
class Car {
private:
string id; // 编号
string model; // 型号
string color; // 颜色
string maker; // 生产厂家
string date; // 出厂日期
double price; // 价格
public:
Car(string id, string model, string color, string maker, string date, double price) {
this->id = id;
this->model = model;
this->color = color;
this->maker = maker;
this->date = date;
this->price = price;
}
string getId() { return id; }
string getModel() { return model; }
string getColor() { return color; }
string getMaker() { return maker; }
string getDate() { return date; }
double getPrice() { return price; }
};
// 定义Employee类
class Employee {
private:
string id; // 员工编号
string name; // 姓名
string gender; // 性别
int age; // 年龄
string hometown;// 籍贯
string education;//学历
public:
Employee(string id, string name, string gender, int age, string hometown, string education) {
this->id = id;
this->name = name;
this->gender = gender;
this->age = age;
this->hometown = hometown;
this->education = education;
}
string getId() { return id; }
string getName() { return name; }
string getGender() { return gender; }
int getAge() { return age; }
string getHometown() { return hometown; }
string getEducation() { return education; }
};
// 定义Customer类
class Customer {
private:
string name; // 客户名称
string contact; // 联系方式
string address; // 地址
vector<string> records; // 业务联系记录
public:
Customer(string name, string contact, string address) {
this->name = name;
this->contact = contact;
this->address = address;
}
string getName() { return name; }
string getContact() { return contact; }
string getAddress() { return address; }
void addRecord(string record) { records.push_back(record); }
vector<string> getRecords() { return records; }
};
// 定义Sales类
class Sales {
private:
string date; // 销售日期
string type; // 汽车类型
string color; // 颜色
int amount; // 数量
string employeeId; // 经手人
public:
Sales(string date, string type, string color, int amount, string employeeId) {
this->date = date;
this->type = type;
this->color = color;
this->amount = amount;
this->employeeId = employeeId;
}
string getDate() { return date; }
string getType() { return type; }
string getColor() { return color; }
int getAmount() { return amount; }
string getEmployeeId() { return employeeId; }
};
// 定义一个全局的vector变量,用于存储汽车信息
vector<Car> cars;
// 定义一个全局的vector变量,用于存储员工信息
vector<Employee> employees;
// 定义一个全局的vector变量,用于存储客户信息
vector<Customer> customers;
// 定义一个全局的vector变量,用于存储销售信息
vector<Sales> sales;
// 添加汽车信息
void addCar() {
string id, model, color, maker, date;
double price;
cout << "请输入汽车编号:";
cin >> id;
cout << "请输入汽车型号:";
cin >> model;
cout << "请输入汽车颜色:";
cin >> color;
cout << "请输入汽车生产厂家:";
cin >> maker;
cout << "请输入汽车出厂日期:";
cin >> date;
cout << "请输入汽车价格:";
cin >> price;
Car car(id, model, color, maker, date, price);
cars.push_back(car);
cout << "添加成功!" << endl;
}
// 添加员工信息
void addEmployee() {
string id, name, gender, hometown, education;
int age;
cout << "请输入员工编号:";
cin >> id;
cout << "请输入员工姓名:";
cin >> name;
cout << "请输入员工性别:";
cin >> gender;
cout << "请输入员工年龄:";
cin >> age;
cout << "请输入员工籍贯:";
cin >> hometown;
cout << "请输入员工学历:";
cin >> education;
Employee employee(id, name, gender, age, hometown, education);
employees.push_back(employee);
cout << "添加成功!" << endl;
}
// 添加客户信息
void addCustomer() {
string name, contact, address;
cout << "请输入客户名称:";
cin >> name;
cout << "请输入客户联系方式:";
cin >> contact;
cout << "请输入客户地址:";
cin >> address;
Customer customer(name, contact, address);
customers.push_back(customer);
cout << "添加成功!" << endl;
}
// 添加销售信息
void addSales() {
string date, type, color, employeeId;
int amount;
cout << "请输入销售日期:";
cin >> date;
cout << "请输入汽车类型:";
cin >> type;
cout << "请输入汽车颜色:";
cin >> color;
cout << "请输入销售数量:";
cin >> amount;
cout << "请输入经手人编号:";
cin >> employeeId;
Sales sale(date, type, color, amount, employeeId);
sales.push_back(sale);
cout << "添加成功!" << endl;
}
// 根据编号查询汽车信息
void findCarById() {
string id;
cout << "请输入汽车编号:";
cin >> id;
bool found = false;
for (auto car : cars) {
if (car.getId() == id) {
cout << "编号:" << car.getId() << endl;
cout << "型号:" << car.getModel() << endl;
cout << "颜色:" << car.getColor() << endl;
cout << "生产厂家:" << car.getMaker() << endl;
cout << "出厂日期:" << car.getDate() << endl;
cout << "价格:" << car.getPrice() << endl;
found = true;
break;
}
}
if (!found) {
cout << "未找到该汽车信息!" << endl;
}
}
// 根据员工姓名查询销售信息
void findSalesByEmployeeName() {
string name;
cout << "请输入员工姓名:";
cin >> name;
bool found = false;
for (auto sale : sales) {
for (auto employee : employees) {
if (sale.getEmployeeId() == employee.getId() && employee.getName() == name) {
cout << "销售日期:" << sale.getDate() << endl;
cout << "汽车类型:" << sale.getType() << endl;
cout << "汽车颜色:" << sale.getColor() << endl;
cout << "销售数量:" << sale.getAmount() << endl;
cout << "经手人姓名:" << employee.getName() << endl;
found = true;
break;
}
}
}
if (!found) {
cout << "未找到该员工的销售信息!" << endl;
}
}
// 输出销售报表
void printSalesReport() {
cout << "销售报表:" << endl;
cout << "销售日期\t汽车类型\t汽车颜色\t销售数量\t经手人姓名" << endl;
for (auto sale : sales) {
for (auto employee : employees) {
if (sale.getEmployeeId() == employee.getId()) {
cout << sale.getDate() << "\t" << sale.getType() << "\t" << sale.getColor() << "\t" << sale.getAmount() << "\t" << employee.getName() << endl;
break;
}
}
}
}
// 主函数
int main() {
int choice;
while (true) {
cout << "请选择操作:" << endl;
cout << "1.添加汽车信息" << endl;
cout << "2.添加员工信息" << endl;
cout << "3.添加客户信息" << endl;
cout << "4.添加销售信息" << endl;
cout << "5.根据编号查询汽车信息" << endl;
cout << "6.根据员工姓名查询销售信息" << endl;
cout << "7.输出销售报表" << endl;
cout << "8.退出系统" << endl;
cin >> choice;
switch (choice) {
case 1:
addCar();
break;
case 2:
addEmployee();
break;
case 3:
addCustomer();
break;
case 4:
addSales();
break;
case 5:
findCarById();
break;
case 6:
findSalesByEmployeeName();
break;
case 7:
printSalesReport();
break;
case 8:
return 0;
default:
cout << "无效的操作!" << endl;
break;
}
}
return 0;
}
```
上述程序实现了添加汽车、员工、客户和销售信息的功能,并提供了根据编号查询汽车信息、根据员工姓名查询销售信息和输出销售报表的功能。您可以根据需要对程序进行修改和扩展。
相关推荐













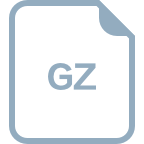
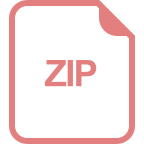
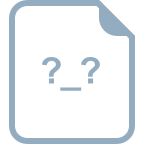