编写程序找出在三维空间上距离最近的两个点,使用一个二维数组存放点的坐标。 要求如下: (1)使用下面的方法头读取用户输入的点的坐标,要求输入6个点; public static double[][] getArray(int n1,int n2); (2)使用下面的方法头计算两个点之间的距离。 public static double distance(double x1, double y1, double z1, double x2, double y2, double z2) (3)使用下面的方法头计算最短距离的两个点及返回最短距离。 public static double getShortestDistance(double[][] points,double[][] shortDistancePoint) 说明: double[][] points 存放三维空间上若干个点; double[][] shortDistancePoint存放最短距离的两个点; (4)使用下面的方法头打印输出最短距离的两个点。 public static void printShortestDistance(double[][] shortDistancePoint)
时间: 2024-03-10 11:50:59 浏览: 68
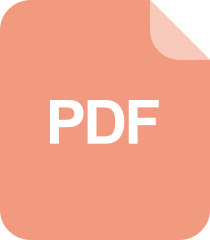
Java 编写Point类,构造方法,计算两个点之间的距离,键盘输入两个点,格式以“,”隔开,如:1,1即为(1,1)点。

好的,这是一个关于计算三维空间上最近距离的问题,下面是我的建议:
```java
import java.util.Scanner;
public class ShortestDistance {
public static void main(String[] args) {
Scanner input = new Scanner(System.in);
double[][] points = getArray(6, 3);
double[][] shortDistancePoint = new double[2][3];
double shortestDistance = getShortestDistance(points, shortDistancePoint);
System.out.println("The shortest distance is " + shortestDistance);
printShortestDistance(shortDistancePoint);
}
public static double[][] getArray(int n1, int n2) {
Scanner input = new Scanner(System.in);
double[][] array = new double[n1][n2];
System.out.println("Enter " + n1 + " points with " + n2 + " coordinates each:");
for (int i = 0; i < n1; i++) {
for (int j = 0; j < n2; j++) {
array[i][j] = input.nextDouble();
}
}
return array;
}
public static double distance(double x1, double y1, double z1, double x2, double y2, double z2) {
return Math.sqrt(Math.pow(x2 - x1, 2) + Math.pow(y2 - y1, 2) + Math.pow(z2 - z1, 2));
}
public static double getShortestDistance(double[][] points, double[][] shortDistancePoint) {
double shortestDistance = Double.MAX_VALUE;
int n = points.length;
for (int i = 0; i < n; i++) {
for (int j = i + 1; j < n; j++) {
double d = distance(points[i][0], points[i][1], points[i][2], points[j][0], points[j][1], points[j][2]);
if (d < shortestDistance) {
shortestDistance = d;
shortDistancePoint[0] = points[i];
shortDistancePoint[1] = points[j];
}
}
}
return shortestDistance;
}
public static void printShortestDistance(double[][] shortDistancePoint) {
System.out.println("The closest two points are (" + shortDistancePoint[0][0] + ", " + shortDistancePoint[0][1] + ", " + shortDistancePoint[0][2] + ") and (" + shortDistancePoint[1][0] + ", " + shortDistancePoint[1][1] + ", " + shortDistancePoint[1][2] + ")");
}
}
```
该程序首先使用 `getArray` 方法读取用户输入的点的坐标,然后使用 `getShortestDistance` 方法计算最短距离的两个点及返回最短距离,接着使用 `printShortestDistance` 方法打印输出最短距离的两个点。其中,`distance` 方法用于计算两个点之间的距离。
注意,该程序假设用户输入的点的数量是 6,每个点的坐标是三维的。如果需要适应其他数量或维度的点,请相应地修改 `getArray` 方法中的参数。
阅读全文
相关推荐
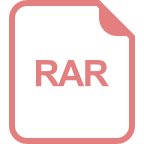
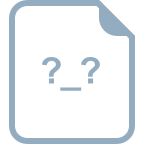
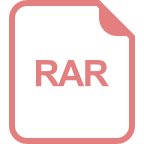
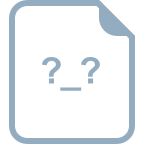
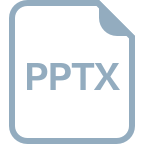
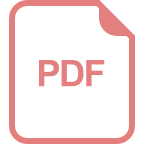
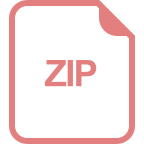
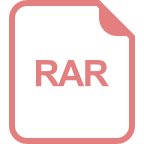
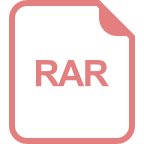