函数create_linkfile5_sl检查./file5文件是否存在,如果存在则创建软链接文件./file5_sl,然后返回 0 。如果不存在,则返回 -1;调用 create_linkfile5_sl, delete_linkfile5_sl, create_linkfile6_sl, delete_linkfile6_sl 函数分别检查 file5 ,file6 是否存在,如果存在,则创建对应的软链接文件: file5 _sl, file6_sl,然后删除。
时间: 2024-02-25 07:58:42 浏览: 73
以下是一个完整的实现,使用C语言的系统调用函数实现:
```
#include <unistd.h>
#include <stdio.h>
#include <errno.h>
int create_linkfile5_sl() {
// 检查文件是否存在
if (access("./file5", F_OK) == -1) {
// 文件不存在,返回-1
return -1;
} else {
// 创建软链接文件
int result = symlink("./file5", "./file5_sl");
if (result == -1) {
// 创建软链接失败,返回错误码
return errno;
} else {
// 创建软链接成功,返回0
return 0;
}
}
}
int delete_linkfile5_sl() {
// 删除软链接文件
int result = unlink("./file5_sl");
if (result == -1) {
// 删除软链接文件失败,返回错误码
return errno;
} else {
// 删除软链接文件成功,返回0
return 0;
}
}
int create_linkfile6_sl() {
// 检查文件是否存在
if (access("./file6", F_OK) == -1) {
// 文件不存在,返回-1
return -1;
} else {
// 创建软链接文件
int result = symlink("./file6", "./file6_sl");
if (result == -1) {
// 创建软链接失败,返回错误码
return errno;
} else {
// 创建软链接成功,返回0
return 0;
}
}
}
int delete_linkfile6_sl() {
// 删除软链接文件
int result = unlink("./file6_sl");
if (result == -1) {
// 删除软链接文件失败,返回错误码
return errno;
} else {
// 删除软链接文件成功,返回0
return 0;
}
}
int main() {
// 创建软链接文件file5_sl
int result = create_linkfile5_sl();
if (result == -1) {
printf("file5 not exist\n");
} else if (result != 0) {
printf("create file5_sl failed with errno %d\n", result);
} else {
printf("create file5_sl success\n");
}
// 删除软链接文件file5_sl
result = delete_linkfile5_sl();
if (result != 0) {
printf("delete file5_sl failed with errno %d\n", result);
} else {
printf("delete file5_sl success\n");
}
// 创建软链接文件file6_sl
result = create_linkfile6_sl();
if (result == -1) {
printf("file6 not exist\n");
} else if (result != 0) {
printf("create file6_sl failed with errno %d\n", result);
} else {
printf("create file6_sl success\n");
}
// 删除软链接文件file6_sl
result = delete_linkfile6_sl();
if (result != 0) {
printf("delete file6_sl failed with errno %d\n", result);
} else {
printf("delete file6_sl success\n");
}
return 0;
}
```
该程序首先调用 `create_linkfile5_sl` 函数创建软链接文件 `file5_sl`,并输出相应的信息。然后调用 `delete_linkfile5_sl` 函数删除软链接文件 `file5_sl`,同样输出相应的信息。接下来调用 `create_linkfile6_sl` 和 `delete_linkfile6_sl` 函数,分别创建和删除软链接文件 `file6_sl`,并输出相应的信息。
阅读全文
相关推荐
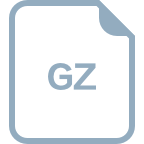




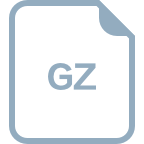



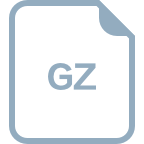





