假设我们有一个Person 对象列表,每个 Person 对象包含 name 和age 两个属性,现在需要按照年龄(age)属性对 Person 对象进行分组,对分组后的数据按照某个属性进行排序,并返回排序后的结果
时间: 2024-03-06 14:49:15 浏览: 40
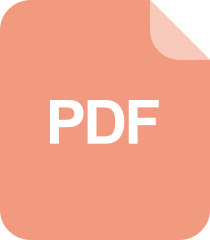
根据对象的某一属性进行排序的js代码(如:name,age)
可以按照以下步骤实现:
1. 使用 Python 中的 groupby 函数按照年龄属性对 Person 对象列表进行分组。示例代码如下:
```python
from itertools import groupby
# 假设 person_list 是 Person 对象列表
person_list = [
{'name': 'Alice', 'age': 20},
{'name': 'Bob', 'age': 25},
{'name': 'Charlie', 'age': 20},
{'name': 'David', 'age': 30},
]
# 按照年龄属性对 Person 对象列表进行分组
grouped_persons = groupby(sorted(person_list, key=lambda x: x['age']), lambda x: x['age'])
# 打印分组后的结果
for age, persons in grouped_persons:
print(f'Age: {age}')
for person in persons:
print(f' Name: {person["name"]}, Age: {person["age"]}')
```
2. 使用 Python 中的 sorted 函数按照某个属性对分组后的数据进行排序。示例代码如下:
```python
# 假设按照年龄属性对 Person 对象进行分组后得到了 grouped_persons 列表
# 对分组后的数据按照 name 属性进行排序,并返回排序后的结果
sorted_persons = []
for age, persons in grouped_persons:
sorted_persons.extend(sorted(persons, key=lambda x: x['name']))
# 打印排序后的结果
for person in sorted_persons:
print(f'Name: {person["name"]}, Age: {person["age"]}')
```
以上示例代码仅供参考,具体实现需要根据实际情况进行调整和优化。
阅读全文
相关推荐
















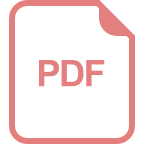