写一段浏览器的插件做ajax请求的代码
时间: 2024-02-09 18:07:03 浏览: 144
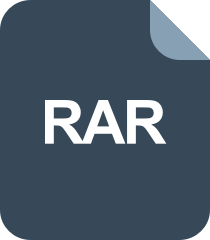
浏览器调试ajax请求
好的,以下是一个简单的Chrome浏览器插件,用于向指定的API发送Ajax请求并将返回结果显示在浏览器界面中:
manifest.json文件:
```json
{
"name": "Ajax Request Plugin",
"version": "1.0",
"description": "This plugin sends Ajax requests and displays the response",
"manifest_version": 2,
"permissions": [
"http://localhost/*",
"https://localhost/*",
"http://example.com/*",
"https://example.com/*",
"activeTab"
],
"background": {
"scripts": ["background.js"],
"persistent": false
},
"browser_action": {
"default_popup": "popup.html"
}
}
```
popup.html文件:
```html
<!DOCTYPE html>
<html>
<head>
<title>Ajax Request Plugin</title>
<script src="popup.js"></script>
</head>
<body>
<h1>Ajax Request Plugin</h1>
<form>
<label for="url">URL:</label>
<input type="text" id="url" name="url" /><br />
<label for="method">Method:</label>
<select id="method" name="method">
<option value="GET">GET</option>
<option value="POST">POST</option>
</select><br />
<label for="data">Data:</label>
<textarea id="data" name="data"></textarea><br />
<input type="button" id="send" value="Send" />
</form>
<div id="response"></div>
</body>
</html>
```
popup.js文件:
```javascript
document.addEventListener("DOMContentLoaded", function() {
var sendButton = document.getElementById("send");
var responseDiv = document.getElementById("response");
sendButton.addEventListener("click", function() {
var url = document.getElementById("url").value;
var method = document.getElementById("method").value;
var data = document.getElementById("data").value;
var xhr = new XMLHttpRequest();
xhr.open(method, url, true);
xhr.setRequestHeader("Content-Type", "application/json");
xhr.onreadystatechange = function() {
if (xhr.readyState == 4 && xhr.status == 200) {
responseDiv.innerHTML = xhr.responseText;
}
};
xhr.send(data);
});
});
```
以上代码中,popup.html是浏览器插件的界面,包含了一个表单用于输入请求的URL、请求方法、请求数据以及一个发送按钮。当用户点击发送按钮时,会触发popup.js中的事件处理函数,创建一个XMLHttpRequest对象,使用指定的URL、方法和数据发送Ajax请求,并将返回结果显示在responseDiv元素中。需要注意的是,插件需要在manifest.json文件中声明所需的权限,否则会限制插件的功能和使用范围。
阅读全文
相关推荐
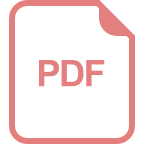
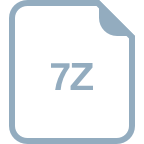
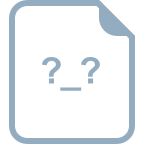
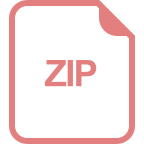
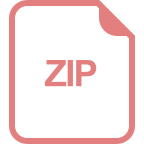
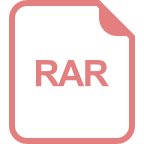
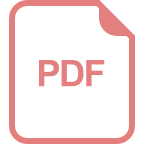
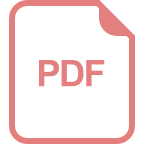
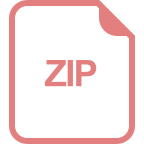
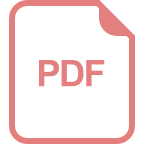
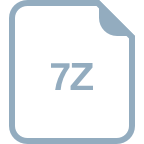
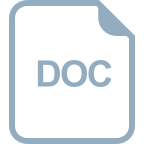
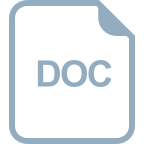
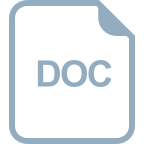
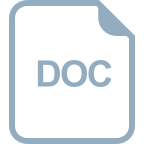
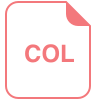
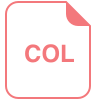