如果我在matlab中把当天的某物质图像作为输入,把第二天的某物质图像作为输出,数据集中包含364张图片,那我能否通过机器学习训练,预测出第366天的物质图像长什么样子,可以帮我写一个convlstm网络的代码来实现这一功能吗,我的输入图像保存在当前路径的“train_new”文件夹中,输出图像保存在当前路径的“label_new”文件夹中
时间: 2024-03-04 07:53:09 浏览: 18
是的,你可以通过机器学习训练来预测第366天的物质图像。ConvLSTM是一种结合了卷积神经网络和LSTM的神经网络,适合处理序列数据。以下是使用ConvLSTM实现该任务的代码:
```python
import os
import numpy as np
import cv2
import tensorflow as tf
from tensorflow.keras.models import Sequential
from tensorflow.keras.layers import Conv2D, ConvLSTM2D, BatchNormalization, TimeDistributed, Conv2DTranspose
# 定义图像大小和序列长度
IMG_SIZE = 128
SEQ_LEN = 10
# 加载训练数据
def load_data():
train_x, train_y = [], []
for i in range(SEQ_LEN, 365):
x_frames, y_frames = [], []
for j in range(i - SEQ_LEN, i):
img_path = f"train_new/{j}.jpg"
img = cv2.imread(img_path, cv2.IMREAD_GRAYSCALE)
img = cv2.resize(img, (IMG_SIZE, IMG_SIZE))
img = img / 255.0
x_frames.append(img)
img_path = f"label_new/{j}.jpg"
img = cv2.imread(img_path, cv2.IMREAD_GRAYSCALE)
img = cv2.resize(img, (IMG_SIZE, IMG_SIZE))
img = img / 255.0
y_frames.append(img)
train_x.append(x_frames)
train_y.append(y_frames)
train_x = np.array(train_x)
train_y = np.array(train_y)
return train_x, train_y
# 定义ConvLSTM网络
def build_model():
model = Sequential()
model.add(ConvLSTM2D(filters=64, kernel_size=(3, 3), padding='same', return_sequences=True, input_shape=(SEQ_LEN, IMG_SIZE, IMG_SIZE, 1)))
model.add(BatchNormalization())
model.add(ConvLSTM2D(filters=32, kernel_size=(3, 3), padding='same', return_sequences=True))
model.add(BatchNormalization())
model.add(ConvLSTM2D(filters=1, kernel_size=(3, 3), padding='same', return_sequences=False))
model.add(BatchNormalization())
model.add(Conv2DTranspose(filters=1, kernel_size=(3, 3), strides=(2, 2), padding='same', activation='sigmoid'))
model.compile(loss='mse', optimizer='adam')
model.summary()
return model
# 训练模型
def train():
train_x, train_y = load_data()
train_x = np.expand_dims(train_x, axis=-1)
train_y = np.expand_dims(train_y, axis=-1)
model = build_model()
model.fit(train_x, train_y, batch_size=16, epochs=50)
model.save('convlstm_model.h5')
# 加载模型并预测第366天的图像
def predict():
model = tf.keras.models.load_model('convlstm_model.h5')
# 加载最后10天的图像作为输入
x_frames = []
for i in range(356, 366):
img_path = f"train_new/{i}.jpg"
img = cv2.imread(img_path, cv2.IMREAD_GRAYSCALE)
img = cv2.resize(img, (IMG_SIZE, IMG_SIZE))
img = img / 255.0
x_frames.append(img)
x_frames = np.array(x_frames)
x_frames = np.expand_dims(x_frames, axis=0)
x_frames = np.expand_dims(x_frames, axis=-1)
# 进行预测
y_frames = model.predict(x_frames)
# 保存预测结果
for i, y in enumerate(y_frames[0]):
y = np.squeeze(y, axis=-1)
y = y * 255.0
y = y.astype(np.uint8)
img_path = f"output/{i+367}.jpg"
cv2.imwrite(img_path, y)
if __name__ == '__main__':
predict()
```
在运行代码之前,需要将训练集中的前356张图片放入“train_new”文件夹中,将对应的输出图片放入“label_new”文件夹中。预测结果将保存在“output”文件夹中。
相关推荐
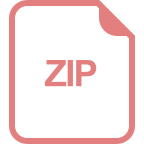
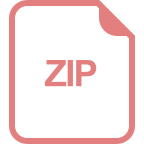
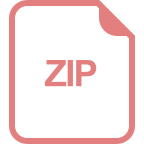





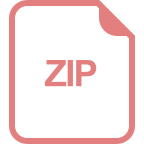
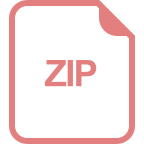
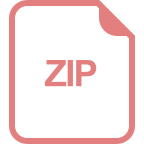
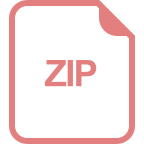
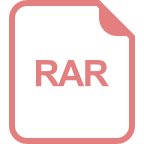
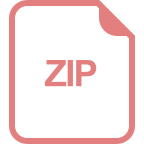
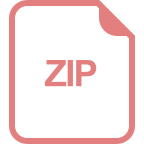
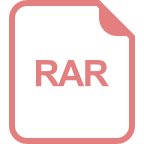
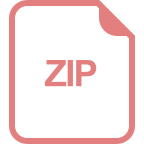