matlab R2019a中,一组三维坐标数据基于麻雀搜索算法(SSA)的三维旅行商问题代码,包括子函数代码
时间: 2024-02-18 12:02:04 浏览: 150
以下是一个基于麻雀搜索算法(SSA)的三维旅行商问题的Matlab代码,其中包括了主函数和子函数代码:
主函数代码:
```matlab
clc;
clear;
close all;
% 随机生成一组三维坐标数据
N = 50; % 城市数量
x = 1000*rand(N,1);
y = 1000*rand(N,1);
z = 1000*rand(N,1);
D = distance(x,y,z); % 计算城市之间的距离矩阵
% 初始化SSA算法的参数
n = 50; % 雀群数量
T = 100; % 迭代次数
a = 2; % 随机步长的上限
r = 0.5; % 随机因子
p = 0.5; % 选择因子
% 运行SSA算法求解三维旅行商问题
[best_path, best_dist] = ssa_tsp_3d(D, n, T, a, r, p, x, y, z);
% 绘制旅行商问题的最优路径
plot_tsp_3d(best_path, x, y, z);
```
子函数代码:
```matlab
function [best_path, best_dist] = ssa_tsp_3d(D, n, T, a, r, p, x, y, z)
% 麻雀搜索算法求解三维旅行商问题
% 输入参数:
% D——城市之间的距离矩阵
% n——雀群数量
% T——迭代次数
% a——随机步长的上限
% r——随机因子
% p——选择因子
% x,y,z——城市的三维坐标
% 输出参数:
% best_path——旅行商问题的最优路径
% best_dist——旅行商问题的最优路径长度
N = size(D,1); % 城市数量
X = zeros(N,n); % 雀群的位置矩阵
V = zeros(N,n); % 雀群的速度矩阵
pBest_X = X; % 雀群的历史最优位置矩阵
Gbest_X = zeros(N,1); % 集群的全局最优位置
Gbest_dist = Inf; % 集群的全局最优路径长度
dist = zeros(n,1); % 雀群的路径长度向量
for i = 1:n
X(:,i) = randperm(N)'; % 随机初始化雀群的位置
dist(i) = get_dist_3d(D,X(:,i),x,y,z); % 计算雀群的路径长度
if dist(i) < Gbest_dist % 更新集群的全局最优位置
Gbest_X = X(:,i);
Gbest_dist = dist(i);
end
end
pBest_X = X; % 初始化雀群的历史最优位置矩阵
for t = 1:T % 开始迭代
for i = 1:n % 更新雀群的速度和位置
r1 = rand(N,1); % 随机因子1
r2 = rand(N,1); % 随机因子2
V(:,i) = r.*V(:,i) + a.*(pBest_X(:,i)-X(:,i)).*r1 + a.*(Gbest_X-X(:,i)).*r2; % 更新速度
X(:,i) = X(:,i) + V(:,i); % 更新位置
X(:,i) = mod(X(:,i)-1,N)+1; % 处理位置越界
dist(i) = get_dist_3d(D,X(:,i),x,y,z); % 计算路径长度
if dist(i) < get_dist_3d(D,pBest_X(:,i),x,y,z) % 更新历史最优位置
pBest_X(:,i) = X(:,i);
end
if dist(i) < Gbest_dist % 更新全局最优位置
Gbest_X = X(:,i);
Gbest_dist = dist(i);
end
end
% 随机选择一些雀群进行位置重置
idx = rand(n,1) < p;
for i = 1:n
if idx(i)
X(:,i) = randperm(N)';
dist(i) = get_dist_3d(D,X(:,i),x,y,z); % 计算路径长度
if dist(i) < get_dist_3d(D,pBest_X(:,i),x,y,z) % 更新历史最优位置
pBest_X(:,i) = X(:,i);
end
if dist(i) < Gbest_dist % 更新全局最优位置
Gbest_X = X(:,i);
Gbest_dist = dist(i);
end
end
end
end
best_path = Gbest_X; % 输出最优路径
best_dist = Gbest_dist; % 输出最优路径长度
end
function dist = get_dist_3d(D,path,x,y,z)
% 计算三维旅行商问题的路径长度
dist = 0;
for i = 1:length(path)-1
dist = dist + D(path(i),path(i+1));
end
dist = dist + D(path(end),path(1));
x_path = x(path);
y_path = y(path);
z_path = z(path);
dist = dist + sum(sqrt(diff(x_path).^2 + diff(y_path).^2 + diff(z_path).^2)));
end
function D = distance(x,y,z)
% 计算三维坐标之间的距离矩阵
N = length(x);
D = zeros(N);
for i = 1:N
for j = 1:N
D(i,j) = sqrt((x(i)-x(j))^2 + (y(i)-y(j))^2 + (z(i)-z(j))^2);
end
end
end
function plot_tsp_3d(path,x,y,z)
% 绘制三维旅行商问题的路径
plot3(x(path),y(path),z(path),'-o','MarkerSize',5,'LineWidth',1);
xlabel('x');
ylabel('y');
zlabel('z');
end
```
阅读全文
相关推荐
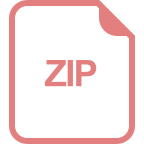
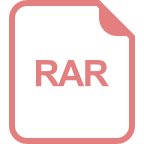
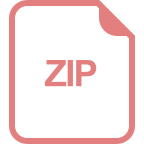
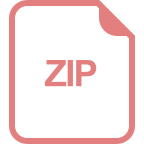
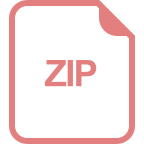
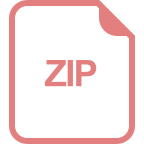
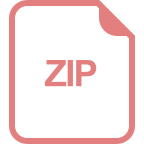
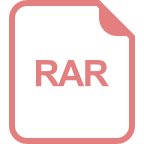
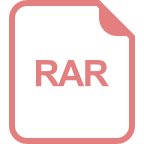
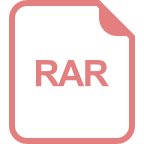
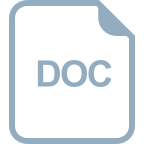
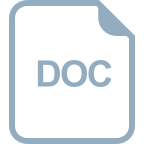


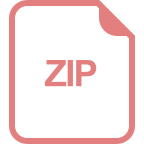

