进行Linux下的文件I/O操作。首先,创建文件test.c;然后使用open()函数打开文件/home/guestuser1/work/file2;接着使用write()函数在文件中写入数据“Hello”;最后使用read()函数将数据读取。
时间: 2024-03-02 14:50:17 浏览: 64
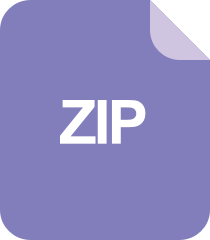
linux 文件I/O编程
好的,我可以帮你回答这个问题。
下面是一个简单的示例程序,演示如何在 Linux 下进行文件 I/O 操作:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <fcntl.h>
int main()
{
char buf[1024];
int fd;
// 创建文件 test.c
fd = open("test.c", O_CREAT | O_TRUNC | O_WRONLY, S_IRUSR | S_IWUSR);
if (fd == -1) {
perror("open");
exit(1);
}
// 打开文件 /home/guestuser1/work/file2
fd = open("/home/guestuser1/work/file2", O_WRONLY);
if (fd == -1) {
perror("open");
exit(1);
}
// 写入数据 "Hello"
if (write(fd, "Hello", 5) == -1) {
perror("write");
exit(1);
}
// 读取数据
lseek(fd, 0, SEEK_SET);
if (read(fd, buf, 5) == -1) {
perror("read");
exit(1);
}
buf[5] = '\0';
printf("Read: %s\n", buf);
// 关闭文件
close(fd);
return 0;
}
```
在这个程序中,我们首先使用 `open()` 函数创建文件 `test.c`,并使用 `O_CREAT` 和 `O_TRUNC` 标志来指定文件不存在时创建文件,文件存在时截断文件(清空文件内容)。然后,我们使用 `open()` 函数打开文件 `/home/guestuser1/work/file2`,并使用 `O_WRONLY` 标志来指定只写模式。接着,我们使用 `write()` 函数在文件中写入数据 "Hello",并使用 `read()` 函数读取数据。最后,我们使用 `close()` 函数关闭文件。
注意,在实际的编程中,我们需要对每个文件操作进行错误检查,并适当地处理错误情况。另外,在 Linux 下进行文件 I/O 操作时,还需要注意文件权限的设置,以确保文件能够被正确地读取和写入。
阅读全文
相关推荐
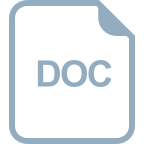


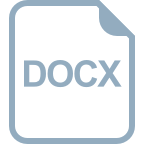
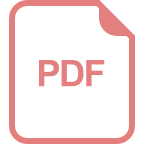
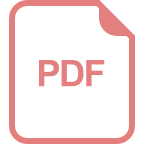
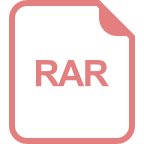
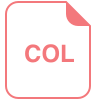
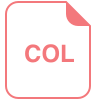
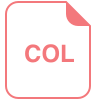
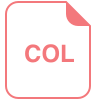
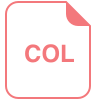
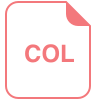
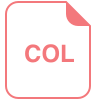

