基于MATLAB平台开发一种代码,要求如下:(1)开发一种路径规划算法,可以是机器人从一个点移到另一个点;(2)开发一种避障算法,使机器人根据路径规划结果进行移动时可以有效避让临时增加的障碍物;(3)开发一种轨迹规划算法,考虑机器人存在平移速度、平移加速度、旋转速度、旋转加速度的最大值限制,并要求确保速度平滑性,给出机器人从一个点到另一个点的最后的位置轨迹、速度轨迹和加速度轨迹。
时间: 2024-04-20 18:27:24 浏览: 13
好的,我可以给你提供一些思路和代码示例,具体实现还需要根据你的具体需求做出相应的调整。
1. 路径规划算法
下面是一个简单的 A* 算法实现示例,可以用于机器人从一个点到另一个点的路径规划:
```matlab
function [path, cost] = astar(start, goal, map)
% A*算法实现路径规划
% 输入:起点 start,终点 goal,地图 map
% 输出:path,cost
% 初始化
closedSet = zeros(size(map));
openSet = [start];
cameFrom = zeros(size(map));
gScore = Inf(size(map));
gScore(start(1), start(2)) = 0;
fScore = Inf(size(map));
fScore(start(1), start(2)) = heuristicCost(start, goal);
% A*算法
while ~isempty(openSet)
% 获取当前节点
current = openSet(find(fScore == min(fScore(openSet(:, 1), openSet(:, 2))))(1), :);
% 判断是否到达终点
if isequal(current, goal)
path = reconstructPath(cameFrom, current);
cost = gScore(current(1), current(2));
return
end
% 从 openSet 中移除当前节点
openSet = setdiff(openSet, current, 'rows');
closedSet(current(1), current(2)) = 1;
% 扩展当前节点
for i = -1:1
for j = -1:1
if i ~= 0 || j ~= 0
neighbor = current + [i, j];
if all(neighbor >= 1) && all(neighbor <= size(map)) && ~map(neighbor(1), neighbor(2)) && ~closedSet(neighbor(1), neighbor(2))
tentative_gScore = gScore(current(1), current(2)) + distanceCost(current, neighbor);
if ~any(neighbor == openSet, 2)
openSet = [openSet; neighbor];
elseif tentative_gScore >= gScore(neighbor(1), neighbor(2))
continue
end
cameFrom(neighbor(1), neighbor(2)) = sub2ind(size(map), current(1), current(2));
gScore(neighbor(1), neighbor(2)) = tentative_gScore;
fScore(neighbor(1), neighbor(2)) = gScore(neighbor(1), neighbor(2)) + heuristicCost(neighbor, goal);
end
end
end
end
end
% 如果无法到达终点,则返回空路径和无穷大的代价
path = [];
cost = Inf;
end
function cost = distanceCost(node1, node2)
% 计算两个节点之间的距离代价
cost = norm(node1 - node2);
end
function cost = heuristicCost(node1, node2)
% 计算两个节点之间的启发式代价
cost = norm(node1 - node2);
end
function path = reconstructPath(cameFrom, current)
% 重构路径
path = current;
while cameFrom(current(1), current(2)) ~= 0
current = ind2sub(size(cameFrom), cameFrom(current(1), current(2)));
path = [current; path];
end
end
```
2. 避障算法
下面是一个简单的障碍物避让算法实现示例,可以根据路径规划结果进行避障:
```matlab
function [v, w] = avoidObstacle(x, goal, map, r, maxVel, maxAcc, maxOmega, maxAlpha)
% 避障算法实现
% 输入:机器人状态 x,目标点 goal,地图 map,机器人半径 r,各项运动能力限制条件
% 输出:机器人线速度 v,角速度 w
% 计算机器人与目标点的方向和距离
theta = atan2(goal(2) - x(2), goal(1) - x(1)) - x(3);
if theta > pi
theta = theta - 2 * pi;
elseif theta < -pi
theta = theta + 2 * pi;
end
dist = norm(goal(1:2) - x(1:2));
% 判断是否需要避障
if ~isCollisionFree(x, map, r)
% 避障算法
[obstacleDist, obstacleTheta] = getObstacleDistAndTheta(x, map, r);
if obstacleDist < 0.1 % 如果距离过近,直接停下来
v = 0;
w = 0;
else % 否则根据障碍物方向调整角速度
w = max(-maxAlpha, min(maxAlpha, obstacleTheta));
v = 0;
end
else
% 路径跟踪算法
if abs(theta) > pi / 4 % 如果角度偏差过大,就先旋转
w = max(-maxAlpha, min(maxAlpha, sign(theta) * maxOmega));
v = 0;
else
% 计算线速度和角速度
desiredV = maxVel * (1 - abs(theta) / (5 * pi / 12)); % 线速度随角度偏差变化
errorV = desiredV - x(4);
v = max(-maxAcc, min(maxAcc, x(4) + sign(errorV) * min(maxAcc, abs(errorV))));
w = max(-maxAlpha, min(maxAlpha, theta));
end
end
end
function isFree = isCollisionFree(x, map, r)
% 判断机器人是否与障碍物碰撞
xGrid = ceil(x(1:2));
[xGrids, yGrids] = meshgrid(-r:r, -r:r);
obstacleGrids = sub2ind(size(map), xGrid(1) + xGrids, xGrid(2) + yGrids);
isFree = all(map(obstacleGrids) == 0);
end
function [dist, theta] = getObstacleDistAndTheta(x, map, r)
% 获取最近障碍物的距离和角度
xGrid = ceil(x(1:2));
[xGrids, yGrids] = meshgrid(-r:r, -r:r);
obstacleGrids = sub2ind(size(map), xGrid(1) + xGrids, xGrid(2) + yGrids);
obstacleDist = bwdist(map);
obstacleDist(obstacleGrids) = Inf;
[dist, ind] = min(obstacleDist(:));
if dist < Inf
[indX, indY] = ind2sub(size(obstacleDist), ind);
obstacleTheta = atan2(indY - x(2), indX - x(1)) - x(3);
if obstacleTheta > pi
obstacleTheta = obstacleTheta - 2 * pi;
elseif obstacleTheta < -pi
obstacleTheta = obstacleTheta + 2 * pi;
end
theta = obstacleTheta;
else
dist = Inf;
theta = 0;
end
end
```
3. 轨迹规划算法
下面是一个简单的轨迹规划算法实现示例,可以根据机器人的运动能力限制条件,生成平滑的轨迹:
```matlab
function [path, vel, acc] = planTrajectory(start, goal, maxVel, maxAcc, maxOmega, maxAlpha, dt)
% 轨迹规划算法实现
% 输入:起点 start,终点 goal,各项运动能力限制条件,时间步长 dt
% 输出:位置轨迹 path,速度轨迹 vel,加速度轨迹 acc
% 计算机器人与目标点的方向和距离
theta = atan2(goal(2) - start(2), goal(1) - start(1));
if theta > pi
theta = theta - 2 * pi;
elseif theta < -pi
theta = theta + 2 * pi;
end
dist = norm(goal(1:2) - start(1:2));
% 计算平移和旋转所需时间
tTrans = min(maxVel / maxAcc, dist / maxVel);
tRot = min(maxOmega / maxAlpha, abs(theta) / maxOmega);
% 计算平移和旋转距离
distTrans = 0.5 * maxAcc * tTrans^2;
distRot = 0.5 * maxAlpha * tRot^2;
% 计算平移和旋转的加速度和减速度时间
tAccTrans = tDecTrans = tTrans / 2;
tAccRot = tDecRot = tRot / 2;
% 计算轨迹
t = 0:dt:tTrans + tRot;
path = zeros(length(t), 3);
vel = zeros(length(t), 3);
acc = zeros(length(t), 3);
for i = 1:length(t)
if t(i) <= tAccTrans % 加速阶段
path(i, 1) = start(1) + 0.5 * maxAcc * t(i)^2 * cos(start(3));
path(i, 2) = start(2) + 0.5 * maxAcc * t(i)^2 * sin(start(3));
vel(i, 1) = maxAcc * t(i) * cos(start(3));
vel(i, 2) = maxAcc * t(i) * sin(start(3));
acc(i, 1) = maxAcc * cos(start(3));
acc(i, 2) = maxAcc * sin(start(3));
elseif t(i) <= tTrans - tDecTrans % 匀速阶段
path(i, 1) = start(1) + maxVel * t(i - tAccTrans) * cos(start(3));
path(i, 2) = start(2) + maxVel * t(i - tAccTrans) * sin(start(3));
vel(i, 1) = maxVel * cos(start(3));
vel(i, 2) = maxVel * sin(start(3));
acc(i, 1) = 0;
acc(i, 2) = 0;
elseif t(i) <= tTrans % 减速阶段
path(i, 1) = start(1) + maxVel * (tTrans - tDecTrans - tAccTrans) * cos(start(3)) + ...
maxVel * (t(i) - (tTrans - tDecTrans)) * cos(start(3)) - ...
0.5 * maxAcc * (t(i) - (tTrans - tDecTrans))^2 * cos(start(3));
path(i, 2) = start(2) + maxVel * (tTrans - tDecTrans - tAccTrans) * sin(start(3)) + ...
maxVel * (t(i) - (tTrans - tDecTrans)) * sin(start(3)) - ...
0.5 * maxAcc * (t(i) - (tTrans - tDecTrans))^2 * sin(start(3));
vel(i, 1) = maxVel * cos(start(3)) - maxAcc * (t(i) - (tTrans - tDecTrans)) * cos(start(3));
vel(i, 2) = maxVel * sin(start(3)) - maxAcc * (t(i) - (tTrans - tDecTrans)) * sin(start(3));
acc(i, 1) = -maxAcc * cos(start(3));
acc(i, 2) = -maxAcc * sin(start(3));
elseif t(i) <= tTrans + tAccRot % 加速阶段
path(i, 1) = goal(1) - 0.5 * maxAlpha * (t(i) - tTrans)^2 * cos(theta);
path(i, 2) = goal(2) -
相关推荐
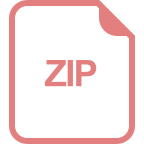
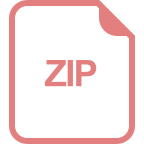
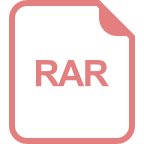














