ESP8266连接阿里云
时间: 2023-11-09 22:15:21 浏览: 296
ESP8266是一款常用的嵌入式Wi-Fi模块,可以用于连接阿里云平台。下面是连接步骤:
1. 在阿里云平台创建一个物联网平台实例,并且创建一个设备。
2. 在ESP8266上安装MQTT库,并且在代码中引入MQTT库。
3. 在代码中配置WIFI连接信息,连接到你的无线网络。
4. 在代码中配置MQTT连接信息,包括阿里云平台的MQTT服务器地址、设备的ProductKey、DeviceName、DeviceSecret等信息。
5. 通过MQTT连接到阿里云平台,进行设备认证和消息订阅。
6. 通过MQTT协议发布和订阅消息,与阿里云平台进行通信。
以下是一个基本的ESP8266连接阿里云的代码示例:
```c
#include <ESP8266WiFi.h>
#include <PubSubClient.h>
const char* ssid = "your_SSID";
const char* password = "your_PASSWORD";
const char* mqtt_server = "your_MQTT_SERVER";
const char* device_id = "your_DEVICE_ID";
const char* product_key = "your_PRODUCT_KEY";
const char* device_secret = "your_DEVICE_SECRET";
const char* sub_topic = "/sys/" + String(product_key) + "/" + String(device_id) + "/thing/service/property/set";
const char* pub_topic = "/sys/" + String(product_key) + "/" + String(device_id) + "/thing/event/property/post";
const char* client_id = "ESP8266Client";
WiFiClient espClient;
PubSubClient client(espClient);
void setup_wifi() {
delay(10);
WiFi.begin(ssid, password);
while (WiFi.status() != WL_CONNECTED) {
delay(500);
}
}
void callback(char* topic, byte* payload, unsigned int length) {
// 处理接收到的消息
}
void reconnect() {
while (!client.connected()) {
String clientId = String(client_id);
if (client.connect(clientId.c_str(), device_id, device_secret)) {
client.subscribe(sub_topic);
} else {
delay(5000);
}
}
}
void setup() {
Serial.begin(9600);
setup_wifi();
client.setServer(mqtt_server, 1883);
client.setCallback(callback);
}
void loop() {
if (!client.connected()) {
reconnect();
}
client.loop();
// 发布消息
String msg = "{\"id\":\"123\",\"params\":{\"switch\":1}}";
client.publish(pub_topic, msg.c_str());
}
```
在以上代码中,需要替换以下变量:
- ssid: 你的Wi-Fi网络名称。
- password: 你的Wi-Fi网络密码。
- mqtt_server: 阿里云平台的MQTT服务器地址。
- device_id: 设备ID,可以在阿里云平台的设备详情页中找到。
- product_key: 产品Key,可以在阿里云平台的产品详情页中找到。
- device_secret: 设备秘钥,可以在阿里云平台的设备详情页中找到。
注意:以上示例代码仅供参考,具体实现根据实际情况进行调整。
阅读全文
相关推荐









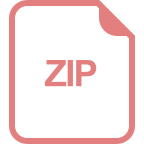
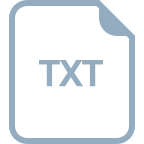