Linux c、c++ 删除,创建,复制文件
时间: 2024-09-07 11:01:05 浏览: 101
在Linux环境下,使用C语言或C++操作文件通常涉及到I/O系统库函数。以下是一些基本操作:
**删除文件**:
- C/C++ (使用`unistd.h`库):
```cpp
#include <unistd.h>
int unlink(const char* filename); // 删除文件
```
例如:
```cpp
unlink("path/to/file");
```
- 错误处理很重要,因为`unlink()`可能会失败,这时可以检查返回值或者使用`errno`。
**创建文件**:
- 使用`open()`和`creat()`函数创建新文件(仅C库):
```cpp
#include <fcntl.h>
#include <unistd.h>
off_t creat(const char *pathname, mode_t mode);
```
例如:
```cpp
int fd = creat("path/to/newfile", S_IRUSR | S_IWUSR | S_IRGRP | S_IROTH);
if (fd == -1) {
perror("Error creating file");
}
```
- C++中可以使用`ofstream`类:
```cpp
#include <fstream>
ofstream file("path/to/newfile");
if (!file) {
std::cerr << "Failed to create file" << std::endl;
}
file.close();
```
**复制文件**:
- 使用C的`rename()`或`copy_file_range()`(从C11开始可用):
```cpp
#include <sys/stat.h>
#include <sys/types.h>
int rename(const char *oldpath, const char *newpath);
// 或者
ssize_t copy_file_range(int out_fd, int in_fd, off_t out_offset, off_t in_offset, size_t count);
```
- C++可以使用`std::ifstream`, `std::ofstream`和`std::copy_n()`:
```cpp
#include <fstream>
std::ifstream in("sourcefile");
std::ofstream out("destinationfile");
std::copy(in.rdbuf()->in(), in.rdbuf()->end(), out.rdbuf());
in.close();
out.close();
```
记得始终处理可能出现的错误和异常。
阅读全文
相关推荐



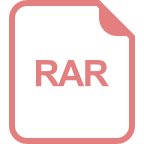

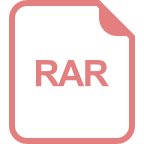










