# 将结果保存为 PNG 文件 with open(output_path, 'wb') as o: # 以二进制写模式打开输出文件。 o.write(output_image) # 将处理后的图像数据写入文件。要求将结果png再转为jpg格式
时间: 2024-10-10 07:05:39 浏览: 13
这个代码片段是用来将图像数据保存为PNG格式的。它首先通过`with`语句以二进制写入模式('wb')打开指定的输出路径`output_path`,这通常是一个文件操作,可以保证文件在操作完成后会被正确关闭。
`o.write(output_image)` 表示将变量`output_image`的内容(即处理后的图像数据)写入到输出文件中,这里假设`output_image`是一个包含图像字节的数据。
如果你需要将PNG转换为JPG格式,你需要先读取PNG文件,然后使用像PIL(Python Imaging Library)这样的库进行转换,最后再次写入到一个新的JPG文件中。以下是简单的步骤:
```python
from PIL import Image
# 假设你已经有一个名为image_data的PNG内容
output_jpg_path = 'output.jpg'
image = Image.open(io.BytesIO(output_image))
image.save(output_jpg_path, 'JPEG')
```
但是上述代码片段本身并不包含这种转换操作,它只是直接保存了PNG数据。
相关问题
使用 input 函数获取读取的字节数; 打印读取的内容; test.png文件的位置为src/step1/test.png; 文件保存的位置为src/step1/file/result.png。用python
在Python中,你可以使用内置的`open()`函数结合`read()`或`readinto()`方法来获取文件内容,并通过`input()`函数配合`os`模块来处理文件路径。下面是一个示例,展示了如何操作`test.png`文件:
```python
import os
import sys
# 定义输入和输出文件路径
file_path = "src/step1/test.png"
output_path = "src/step1/file/result.png"
# 使用二进制模式打开文件以读取字节
with open(file_path, 'rb') as file:
# 使用 input() 获取文件大小 (如果使用的是命令行交互)
bytes_to_read = int(input("请输入要读取的字节数(如果不指定则读取整个文件):"))
if bytes_to_read > 0:
byte_data = file.read(bytes_to_read)
else:
byte_data = file.read()
# 如果需要,打印读取的字节数和内容
print(f"Read {len(byte_data)} bytes from the file.")
print("File content:", byte_data)
# 将读取的内容写入到另一个文件
with open(output_path, 'wb') as output_file:
output_file.write(byte_data)
print(f"Data has been saved to {output_path}.")
现有第一个文件内所有图片的目标物的多边形坐标(在第二个文件夹内)和目标物的二进制掩码图像(在mask文件夹中)如何才能得到文件夹内图片中目标物的xml形式的实例分割标签并保存到第三个文件夹中(完整代码)
这个问题涉及到一些计算机视觉和数据处理方面的知识,需要使用一些库和工具来完成。以下是一个可能的解决方案,需要使用OpenCV、numpy、lxml等库。
```python
import os
import cv2
import numpy as np
from lxml import etree
# 定义数据路径
img_dir = "/path/to/images"
poly_dir = "/path/to/polygons"
mask_dir = "/path/to/masks"
out_dir = "/path/to/output"
# 获取所有图片的文件名
img_files = sorted(os.listdir(img_dir))
# 遍历每张图片
for img_file in img_files:
# 读取图片
img_path = os.path.join(img_dir, img_file)
img = cv2.imread(img_path)
# 获取多边形坐标
poly_path = os.path.join(poly_dir, img_file[:-4] + ".txt")
with open(poly_path, "r") as f:
lines = f.readlines()
polygons = []
for line in lines:
pts = []
coords = line.strip().split(",")
for i in range(0, len(coords), 2):
pts.append((int(coords[i]), int(coords[i+1])))
polygons.append(pts)
# 获取二进制掩码图像
mask_path = os.path.join(mask_dir, img_file[:-4] + ".png")
mask = cv2.imread(mask_path, cv2.IMREAD_GRAYSCALE)
# 将多边形坐标和掩码图像转换为xml形式
root = etree.Element("annotation")
filename = etree.SubElement(root, "filename")
filename.text = img_file
size = etree.SubElement(root, "size")
width = etree.SubElement(size, "width")
width.text = str(img.shape[1])
height = etree.SubElement(size, "height")
height.text = str(img.shape[0])
depth = etree.SubElement(size, "depth")
depth.text = str(img.shape[2])
for i, polygon in enumerate(polygons):
object = etree.SubElement(root, "object")
name = etree.SubElement(object, "name")
name.text = "object{}".format(i+1)
mask_poly = np.zeros_like(mask)
cv2.fillPoly(mask_poly, [np.array(polygon)], 255)
mask_poly = mask_poly & mask
mask_data = etree.SubElement(object, "mask_data")
mask_data.text = np.array2string(mask_poly, separator="", formatter={"int": lambda x: str(x)})
for pt in polygon:
point = etree.SubElement(object, "point")
x = etree.SubElement(point, "x")
x.text = str(pt[0])
y = etree.SubElement(point, "y")
y.text = str(pt[1])
# 保存xml文件
out_path = os.path.join(out_dir, img_file[:-4] + ".xml")
with open(out_path, "wb") as f:
f.write(etree.tostring(root, pretty_print=True))
```
这个代码会遍历所有图片,读取多边形坐标和掩码图像,将它们转换为xml形式的实例分割标签,并保存到输出文件夹中。其中,多边形坐标存储在以图片文件名为名的txt文件中,每行表示一个多边形,每个多边形的顶点坐标以逗号分隔;掩码图像存储在以图片文件名为名的png文件中,每个像素值为0或255,表示是否属于目标物。
阅读全文
相关推荐
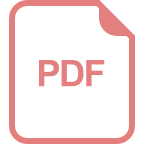
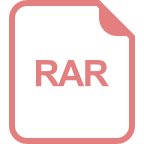
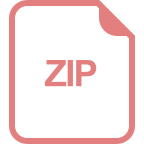
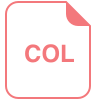
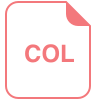
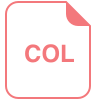
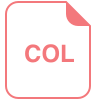
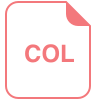
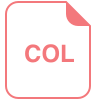
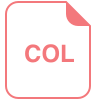
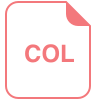
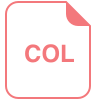
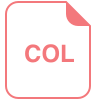
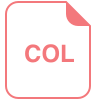
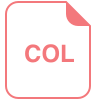
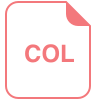