用C++编写一个用单链表实现集合的交集运算的程序。 已知两个集合A和B,现要求一个新的集合A=A∩B。例如,设 A={7,5,3,11},B={2,6,3} 则A和B的交集为 A={3}
时间: 2024-10-26 21:16:29 浏览: 21
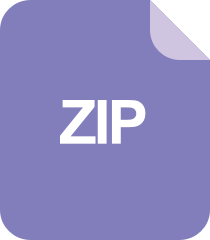
快速四边形交集:计算任意两个四边形的相交面积。-matlab开发
在C++中,我们可以使用STL库中的set数据结构来模拟集合的行为,并利用其自动去重和保持元素有序的特点来进行交集运算。以下是创建一个简单的单链表交集算法的示例:
```cpp
#include <iostream>
#include <unordered_set> // 使用无序集合作为底层存储
using namespace std;
// 链表节点结构体
struct ListNode {
int val;
ListNode* next;
ListNode(int x) : val(x), next(NULL) {}
};
// 将集合转换为链表
ListNode* toLinkedList(set<int>& s) {
ListNode* head = new ListNode(0);
ListNode* tail = head;
for (int i : s) {
tail->next = new ListNode(i);
tail = tail->next;
}
return head->next;
}
// 计算链表交集
ListNode* intersect(ListNode* listA, ListNode* listB) {
set<int> sB(listB->val, listB->next); // 存储列表B的所有元素
ListNode* intersection = nullptr;
ListNode* tempA = listA;
while (tempA != nullptr) {
if (sB.count(tempA->val)) { // 如果A中有B中存在的元素
if (!intersection) { // 创建新的交集链头
intersection = new ListNode(tempA->val);
}
sB.erase(tempA->val); // 从B的集合中移除该元素
}
tempA = tempA->next;
}
return intersection;
}
// 主函数演示
int main() {
set<int> A({7, 5, 3, 11});
set<int> B({2, 6, 3});
ListNode* listA = toLinkedList(A);
ListNode* listB = toLinkedList(B);
ListNode* result = intersect(listA, listB);
cout << "Intersection of A and B as a linked list: ";
while (result != nullptr) {
cout << result->val << " -> ";
result = result->next;
}
cout << "nullptr\n";
return 0;
}
```
这个程序首先将集合A和B分别转换为链表,然后通过遍历列表A并检查元素是否在集合B中来找到它们的交集。最后,它返回一个新的链表,其中包含A和B的公共元素。
阅读全文
相关推荐
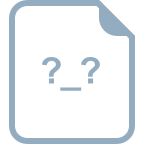
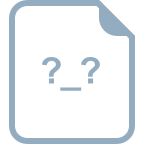
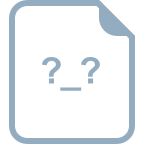
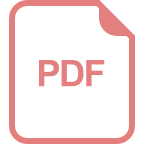
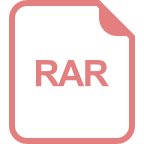
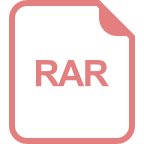
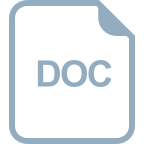
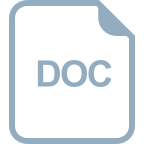
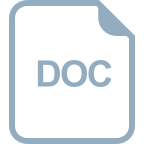
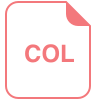
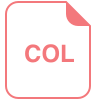
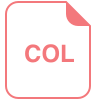
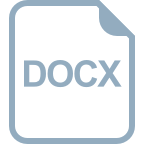
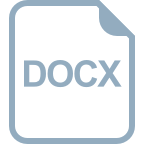