C# csv读取转字典
时间: 2024-11-15 12:24:18 浏览: 14
在C#中,CSV(逗号分隔值)文件读取并转换成字典通常会涉及到使用内置的`System.IO`和`System.Collections.Generic`命名空间里的类。以下是一个基本的步骤示例:
```csharp
using System;
using System.IO;
using System.Collections.Generic;
public class Program
{
public static void Main(string[] args)
{
// 定义CSV文件路径
string filePath = "path_to_your_csv_file.csv";
// 创建一个 Dictionary 对象,键值对将是 CSV 文件的第一行标题
Dictionary<string, string> dictionary = new Dictionary<string, string>();
try
{
using (var reader = new StreamReader(filePath))
{
// 跳过表头
reader.ReadLine();
// 使用 while 循环逐行读取 CSV
while (!reader.EndOfStream)
{
var line = reader.ReadLine();
var values = line.Split(',');
if (dictionary.ContainsKey(values[0])) // 如果键已存在,则覆盖旧值
dictionary[values[0]] = values[1];
else
dictionary.Add(values[0], values[1]); // 否则添加新的键值对
}
}
}
catch (Exception ex)
{
Console.WriteLine($"Error reading CSV file: {ex.Message}");
}
// 输出或进一步处理字典内容
foreach (KeyValuePair<string, string> entry in dictionary)
{
Console.WriteLine($"{entry.Key}: {entry.Value}");
}
}
}
```
在这个例子中,我们首先创建一个空的 `Dictionary`,然后从CSV文件读取每一行,并将第二列的数据作为值,第一列的内容作为对应的键添加到字典中。
阅读全文
相关推荐
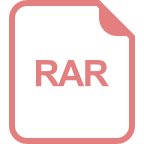
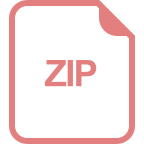
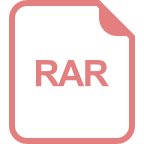


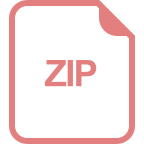
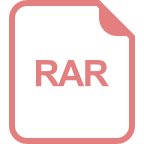
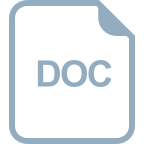
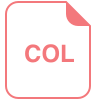








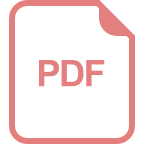