一 实验目的 熟悉掌握启发式搜索算法A*及其可采纳性 二 实验内容 编写程序实现8数码和15数码问题,采用至少两种估价函数 分析估价函数求解问题时候的效率差别,分析估价函数对搜索算法的影响 给我python代码
时间: 2024-11-10 15:13:45 浏览: 50
在Python中,你可以通过实现A*算法来解决八皇后问题(8-puzzle)和十五 puzzle。这里是一个基本的A*算法框架的概述,包括启发式函数(如曼哈顿距离)的使用。请注意,这只是一个简化的版本,实际实现可能需要更多的错误检查和优化。
```python
# 导入必要的模块
from heapq import heappop, heappush
import numpy as np
def heuristic(state, goal):
# 曼哈顿距离作为启发式函数
return sum(abs(a - b) for a, b in zip(state, goal))
def astar_search(start_state, end_state, h=None):
if h is None:
# 如果未提供估价函数,使用默认的曼哈顿距离
h = lambda state: heuristic(state, end_state)
open_set = [(0, start_state)] # 开放集合,包含代价和状态
came_from = {} # 记录每个状态是怎么来的
cost_so_far = {start_state: 0} # 路径成本到当前节点
while open_set:
_, current_state = heappop(open_set)
if current_state == end_state:
break
for next_state, cost in get_neighbors(current_state):
new_cost = cost_so_far[current_state] + cost
if next_state not in cost_so_far or new_cost < cost_so_far[next_state]:
cost_so_far[next_state] = new_cost
priority = new_cost + h(next_state)
heappush(open_set, (priority, next_state))
came_from[next_state] = current_state
path = [end_state]
while end_state != start_state:
end_state = came_from[end_state]
path.append(end_state)
return path[::-1], cost_so_far[end_state]
def get_neighbors(state):
# 这里你需要根据你的问题结构定义邻居的状态生成函数
# 对于8-puzzle,它可能涉及到交换相邻的两个数字位置
pass
# 使用示例
start_state = np.array([4, 0, 7, ..., 6]) # 你的起始状态,根据问题调整
end_state = np.array([0, 1, 2, ..., 9]) # 目标状态
solution, total_cost = astar_search(start_state, end_state)
```
阅读全文
相关推荐
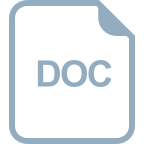
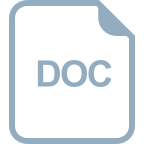
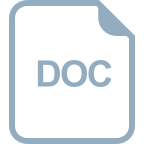
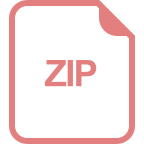
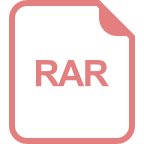
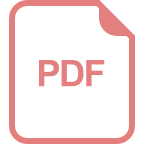
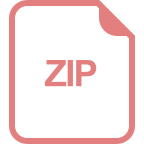
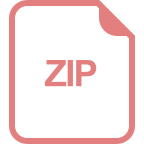
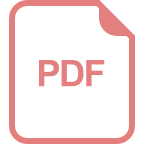
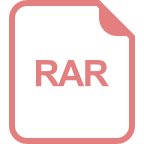
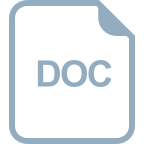
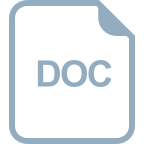
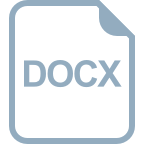