压缩要求对short、float字段都只能用一条语句整体写入,用strcpy实现串的写入。
时间: 2024-09-29 19:04:17 浏览: 31
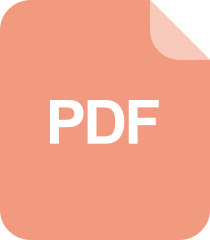
C语言实现字符串操作函数的实例
在C语言中,由于`short`和`float`类型的值无法直接通过`strcpy`(用于字符串的拷贝)函数进行整体写入,因为它们不是字符型。但是我们可以将这两个值转换成字符串格式后再一起写入。
对于`short`,可以先将其转换为十进制字符串,比如用`itoa`函数;对于`float`,可以四舍五入后用`sprintf`或者`snprintf`函数将它转换为字符串加上特定的标识(如`.`和精度位数)。
下面是一个简单的示例,展示了如何做到这一点:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
// 将short整型转换为字符串
char* short_to_str(short value) {
static char buffer[6]; // 预计short的最大范围是32767, 用5位数字表示足够了,加上一位小数点和终止符
sprintf(buffer, "%hd", value);
return buffer;
}
// 将float转换为字符串,保留两位小数
char* float_to_str(float value) {
static char buffer[11];
snprintf(buffer, sizeof(buffer), "%.2f", value);
return buffer;
}
void compress(short s, float f, char* result) {
size_t len = 0;
// 拼接short和float为字符串,中间用特定分隔符
strcat(result, short_to_str(s));
strcat(result, "_");
strcat(result, float_to_str(f));
len = strlen(result);
// 添加终止符
result[len++] = '\0';
}
int main() {
short short_value = 1234;
float float_value = 3.14159;
char compressed[64]; // 假设结果不超过64字节
compress(short_value, float_value, compressed);
printf("Compressed data: %s\n", compressed);
return 0;
}
```
在这个例子中,`compress`函数会将`short`和`float`转换成字符串形式并合并,然后返回整个字符串。注意这只是一个简化版本,实际应用可能需要处理更复杂的情况,比如错误检查和性能优化。
阅读全文
相关推荐
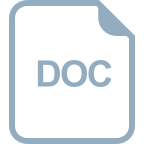
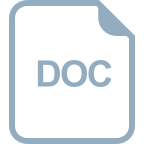

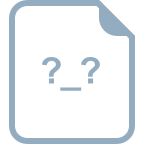
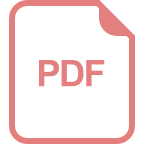
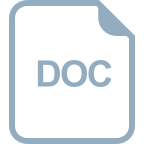
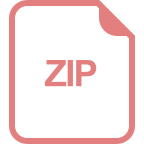
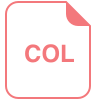






