快速排序算法java 语言
时间: 2024-08-02 12:01:32 浏览: 52
快速排序是一种常用的高效排序算法,它的基本思想是通过一趟排序将待排记录分隔成独立的两部分,其中一部分记录的关键字均比另一部分的关键字小,然后分别对这两部分记录继续进行排序,直到整个序列有序。在Java中,快速排序可以使用递归的方式实现。下面是一个简单的快速排序示例:
```java
public class QuickSort {
public void quickSort(int[] arr, int low, int high) {
if (low < high) {
// 找到划分点
int pivotIndex = partition(arr, low, high);
// 对左右两侧的子数组进行递归排序
quickSort(arr, low, pivotIndex - 1); // 左侧子数组
quickSort(arr, pivotIndex + 1, high); // 右侧子数组
}
}
private int partition(int[] arr, int low, int high) {
int pivot = arr[high]; // 最大值作为基准
int i = (low - 1); // 小于基准的元素索引
for (int j = low; j <= high - 1; j++) {
// 如果当前元素小于等于基准,交换并移动指针
if (arr[j] <= pivot) {
i++;
swap(arr, i, j);
}
}
swap(arr, i + 1, high); // 将基准放在正确的位置
return i + 1;
}
private void swap(int[] arr, int i, int j) {
int temp = arr[i];
arr[i] = arr[j];
arr[j] = temp;
}
}
```
要使用这个排序方法,你可以创建一个`QuickSort`对象并传入你的整数数组:
```java
int[] numbers = {9, 7, 5, 11, 12, 2};
QuickSort qs = new QuickSort();
qs.quickSort(numbers, 0, numbers.length - 1);
System.out.println(Arrays.toString(numbers)); // 输出排序后的数组
阅读全文
相关推荐
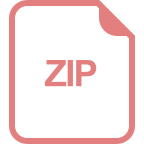
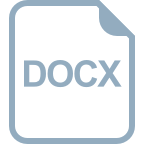
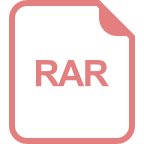
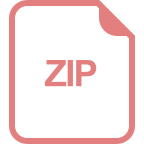
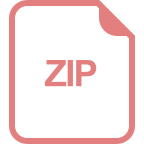
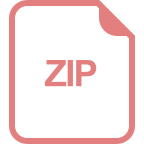
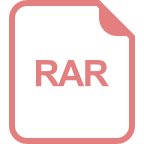
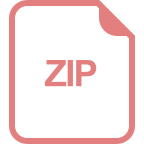
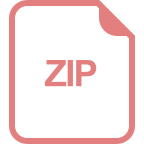
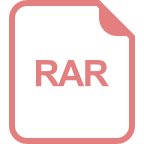
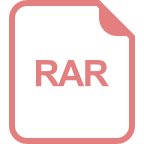
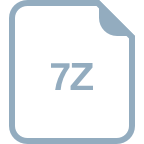
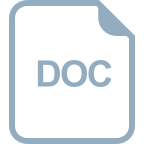
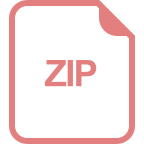