map集合排序 Java
时间: 2024-08-21 20:01:58 浏览: 44
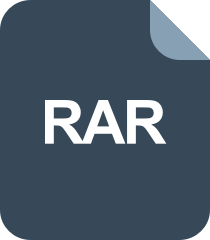
java中Map集合的排序方法
在Java中,Map集合本身是无序的,它不保持元素的添加顺序。但是,我们可以使用不同的方法来对Map中的元素进行排序。最常见的做法是利用Java 8引入的Stream API或者使用传统的方法结合Collection框架来实现。
使用Java 8的Stream API对Map进行排序的常见方式有:
1. 根据键排序(自然排序或自定义排序)
```java
import java.util.*;
import java.util.stream.*;
Map<String, Integer> unsortedMap = new HashMap<>();
unsortedMap.put("apple", 5);
unsortedMap.put("orange", 10);
unsortedMap.put("banana", 7);
// 根据键自然排序
Map<String, Integer> sortedByKey = unsortedMap.entrySet()
.stream()
.sorted(Map.Entry.comparingByKey())
.collect(Collectors.toMap(
Map.Entry::getKey,
Map.Entry::getValue,
(e1, e2) -> e1,
LinkedHashMap::new
));
// 根据键自定义排序
Map<String, Integer> sortedByCustomKey = unsortedMap.entrySet()
.stream()
.sorted((e1, e2) -> e1.getKey().compareToIgnoreCase(e2.getKey()))
.collect(Collectors.toMap(
Map.Entry::getKey,
Map.Entry::getValue,
(e1, e2) -> e1,
LinkedHashMap::new
));
```
2. 根据值排序
```java
// 根据值自然排序
Map<String, Integer> sortedByValue = unsortedMap.entrySet()
.stream()
.sorted(Map.Entry.comparingByValue())
.collect(Collectors.toMap(
Map.Entry::getKey,
Map.Entry::getValue,
(e1, e2) -> e1,
LinkedHashMap::new
));
// 根据值自定义排序
Map<String, Integer> sortedByCustomValue = unsortedMap.entrySet()
.stream()
.sorted((e1, e2) -> e1.getValue().compareTo(e2.getValue()))
.collect(Collectors.toMap(
Map.Entry::getKey,
Map.Entry::getValue,
(e1, e2) -> e1,
LinkedHashMap::new
));
```
使用传统的迭代和比较器来对Map进行排序也是可行的,但相比Stream API来说,代码可能更加繁琐。
阅读全文
相关推荐
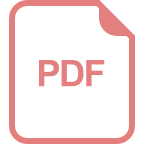
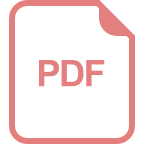
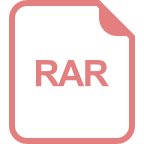
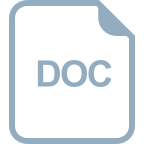
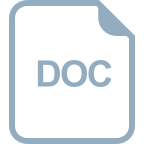
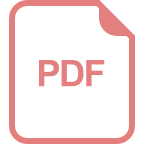
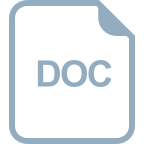
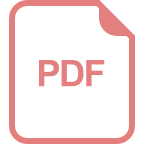
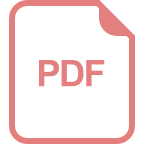
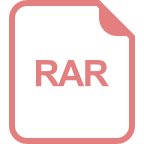
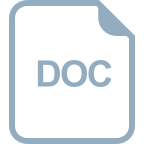
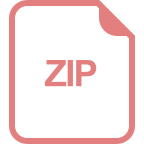
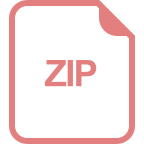
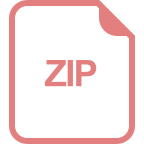
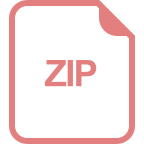