C++获取当前工作目录,并设置指定目录为当前工作目录的代码
时间: 2024-04-23 10:27:46 浏览: 23
要获取当前工作目录并设置指定目录为当前工作目录,你可以使用C++标准库中的`<filesystem>`头文件(C++17及更高版本)或`<unistd.h>`头文件(旧版本)。
以下是两种方法的示例代码:
使用`<filesystem>`头文件(C++17及更高版本):
```cpp
#include <iostream>
#include <filesystem>
namespace fs = std::filesystem;
int main() {
// 获取当前工作目录
std::string currentPath = fs::current_path().string();
std::cout << "当前工作目录:" << currentPath << std::endl;
// 设置指定目录为当前工作目录
std::string newDirectory = "/path/to/new/directory";
fs::current_path(newDirectory);
// 再次获取当前工作目录,确认是否设置成功
currentPath = fs::current_path().string();
std::cout << "新的当前工作目录:" << currentPath << std::endl;
return 0;
}
```
使用`<unistd.h>`头文件(旧版本):
```cpp
#include <iostream>
#include <unistd.h>
int main() {
// 获取当前工作目录
char currentPath[FILENAME_MAX];
if (getcwd(currentPath, sizeof(currentPath)) != nullptr) {
std::cout << "当前工作目录:" << currentPath << std::endl;
} else {
std::cout << "无法获取当前工作目录" << std::endl;
return 1;
}
// 设置指定目录为当前工作目录
const char* newDirectory = "/path/to/new/directory";
if (chdir(newDirectory) == 0) {
std::cout << "已将目录更改为:" << newDirectory << std::endl;
} else {
std::cout << "无法将目录更改为:" << newDirectory << std::endl;
return 1;
}
// 再次获取当前工作目录,确认是否设置成功
if (getcwd(currentPath, sizeof(currentPath)) != nullptr) {
std::cout << "新的当前工作目录:" << currentPath << std::endl;
} else {
std::cout << "无法获取当前工作目录" << std::endl;
return 1;
}
return 0;
}
```
你可以根据你的编译环境和需求选择使用哪种方法。这些代码会获取当前工作目录并显示出来,然后将指定目录设置为当前工作目录,再次获取当前工作目录以确认是否设置成功。
相关推荐
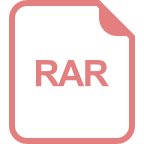
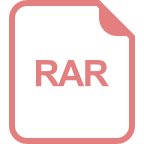














