输入年和月,格式为年\月。输出该月的天数。
时间: 2024-11-29 20:33:47 浏览: 7
在C语言中,为了获取特定月份的天数,我们可以利用内建的日期库函数`strftime()`和`mktime()`。然而,标准库并不直接提供获取单个月份天数的功能,所以我们通常需要编写一些额外的逻辑。下面是一个基于`calendar`库的简单示例,它假设用户输入的是公历的合法年月:
```c
#include <stdio.h>
#include <time.h>
#include <stdlib.h>
#include <string.h>
#include <stdbool.h>
#include <ctype.h> // 用于判断字符是否为数字
size_t getMonthDays(char input[]) {
struct tm date;
time_t timestamp;
memset(&date, 0, sizeof(date));
// 解析输入的年月字符串
char* yearEnd = strtok(input, "-"); // 分割年份和月份
date.tm_year = atoi(yearEnd) - 1900; // 减去1900,转换为系统时间格式
yearEnd = strtok(NULL, "-"); // 获取月份
date.tm_mon = atoi(yearEnd); // 转换为月份(从0开始计)
// 检查输入是否有效
if (!yearEnd || date.tm_mon < 0 || date.tm_mon > 11) {
fprintf(stderr, "Invalid month format. Please enter in the format YYYY-MM.\n");
exit(EXIT_FAILURE);
}
// 设置其他tm结构体成员为默认值
date.tm_mday = 1; // 设置默认日子为1
date.tm_hour = date.tm_min = date.tm_sec = 0;
date.tm_isdst = -1; // 让 mktime 自动处理夏令时
// 将日期转换为时间戳
timestamp = mktime(&date);
// 如果能成功转换,说明输入有效,返回对应的天数
if (timestamp != -1) {
return calendar[1][date.tm_mon]; // 使用 calendar 数组获取对应月份的天数
} else {
fprintf(stderr, "Failed to convert the date. Check the validity of the input.\n");
exit(EXIT_FAILURE);
}
}
int main() {
char input[6];
printf("Enter the date (YYYY-MM): ");
fgets(input, sizeof(input), stdin);
input[strcspn(input, "\n")] = '\0'; // 移除末尾的换行符
size_t days_in_month = getMonthDays(input);
printf("There are %zu days in the specified month.\n", days_in_month);
return 0;
}
// 这里 calendar 数组只是一个示例,实际应用中你需要根据实际的历法数据填充
// calendar[0]通常是月份名称,calendar[1]是每个月的天数
// 注意这个例子只适用于公历,对于其他历法如农历,你需要使用更复杂的算法或第三方库。
阅读全文
相关推荐
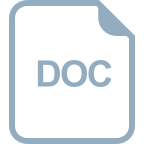
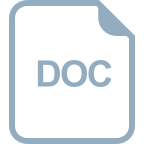
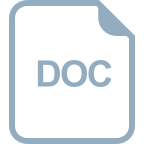















