请帮我用c++语言,二维数组的形式写出贪吃蛇小游戏,包括函数void gotoxy(int x, int y)//光标移动到(x,y)位置 { HANDLE handle = GetStdHandle(STD_OUTPUT_HANDLE); COORD pos; pos.X = x; pos.Y = y; SetConsoleCursorPosition(handle, pos); },
时间: 2024-03-14 14:45:43 浏览: 78
好的,我可以帮你写出一个简单的贪吃蛇游戏,包含函数`gotoxy`,以下是完整代码:
```cpp
#include <iostream>
#include <conio.h>
#include <windows.h>
#include <time.h>
using namespace std;
const int width = 20; // 地图宽度
const int height = 20; // 地图高度
int snakeX, snakeY; // 蛇头坐标
int fruitX, fruitY; // 食物坐标
int tailX[100], tailY[100]; // 蛇身坐标
int nTail; // 蛇身长度
enum Direction { STOP = 0, LEFT, RIGHT, UP, DOWN }; // 方向枚举
Direction dir; // 当前方向
bool gameOver; // 游戏是否结束
void Setup();
void Draw();
void Input();
void Logic();
void gotoxy(int x, int y);
int main()
{
Setup();
while (!gameOver)
{
Draw();
Input();
Logic();
Sleep(50); // 控制游戏速度
}
return 0;
}
void Setup()
{
srand(time(NULL)); // 初始化随机数种子
gameOver = false;
dir = STOP;
snakeX = width / 2; // 蛇头初始坐标
snakeY = height / 2;
fruitX = rand() % width; // 食物初始坐标
fruitY = rand() % height;
nTail = 0;
}
void Draw()
{
system("cls"); // 清屏
for (int i = 0; i < width + 2; i++)
cout << "#"; // 上边框
cout << endl;
for (int i = 0; i < height; i++)
{
for (int j = 0; j < width; j++)
{
if (j == 0)
cout << "#"; // 左边框
if (i == snakeY && j == snakeX)
cout << "O"; // 蛇头
else if (i == fruitY && j == fruitX)
cout << "F"; // 食物
else
{
bool printTail = false;
for (int k = 0; k < nTail; k++)
{
if (tailX[k] == j && tailY[k] == i)
{
cout << "o"; // 蛇身
printTail = true;
}
}
if (!printTail)
cout << " ";
}
if (j == width - 1)
cout << "#"; // 右边框
}
cout << endl;
}
for (int i = 0; i < width + 2; i++)
cout << "#"; // 下边框
cout << endl;
}
void Input()
{
if (_kbhit()) // 判断有没有按键输入
{
switch (_getch())
{
case 'a':
dir = LEFT;
break;
case 'd':
dir = RIGHT;
break;
case 'w':
dir = UP;
break;
case 's':
dir = DOWN;
break;
case 'x':
gameOver = true;
break;
}
}
}
void Logic()
{
int prevX = tailX[0], prevY = tailY[0];
int prev2X, prev2Y;
tailX[0] = snakeX;
tailY[0] = snakeY;
for (int i = 1; i < nTail; i++)
{
prev2X = tailX[i];
prev2Y = tailY[i];
tailX[i] = prevX;
tailY[i] = prevY;
prevX = prev2X;
prevY = prev2Y;
}
switch (dir)
{
case LEFT:
snakeX--;
break;
case RIGHT:
snakeX++;
break;
case UP:
snakeY--;
break;
case DOWN:
snakeY++;
break;
}
// 判断是否撞墙或者碰到自己的身体
if (snakeX < 0 || snakeX >= width || snakeY < 0 || snakeY >= height)
gameOver = true;
for (int i = 0; i < nTail; i++)
{
if (tailX[i] == snakeX && tailY[i] == snakeY)
gameOver = true;
}
// 判断是否吃到了食物
if (snakeX == fruitX && snakeY == fruitY)
{
nTail++;
fruitX = rand() % width;
fruitY = rand() % height;
}
}
void gotoxy(int x, int y)
{
HANDLE handle = GetStdHandle(STD_OUTPUT_HANDLE);
COORD pos;
pos.X = x;
pos.Y = y;
SetConsoleCursorPosition(handle, pos);
}
```
注意:在 Windows 环境下,`gotoxy` 函数需要用到 Windows API,所以需要包含 `windows.h` 头文件。`_kbhit()` 和 `_getch()` 函数需要用到 `conio.h` 头文件,用来实现按键监听。`Sleep()` 函数需要用到 `time.h` 头文件,用来控制游戏速度。
阅读全文
相关推荐
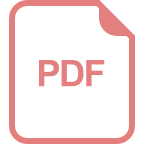
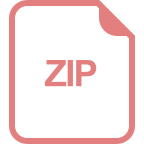
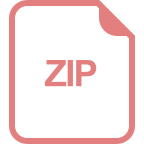

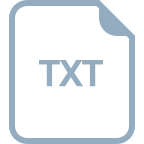
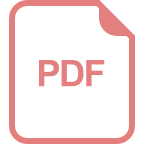
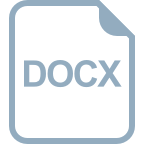
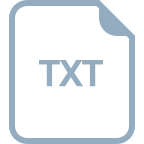
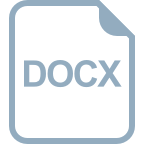
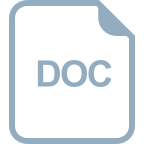
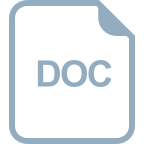
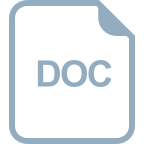
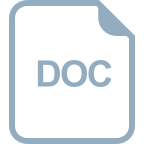
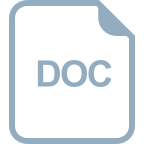
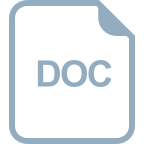
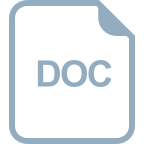
