帮我写一个springboot的banner文字内容是LEMMER-CHAT
时间: 2024-03-19 14:42:01 浏览: 50
当你启动Spring Boot应用程序时,可以自定义一个漂亮的启动横幅。以下是一个简单的示例,可以将其添加到你的应用程序的“src/main/resources/banner.txt”文件中:
```
_____ _____ _
/ ____| / ____| | |
| (___ _ __ ___ _ __| | ___ _ __| |_
\___ \| '_ \ / _ \| '__| | / _ \ '__| __|
____) | |_) | (_) | | | |___| __/ | | |_
|_____/| .__/ \___/|_| \_____\___|_| \__|
| |
|_|
LEMMER-CHAT is starting up...
```
你可以将其复制并粘贴到你的“banner.txt”文件中,然后重新启动你的应用程序,以查看自定义的启动横幅。
相关问题
帮我写一个用python做的智能聊天机器人
当然可以!下面是一个简单的 Python 智能聊天机器人的代码示例,它使用了自然语言处理库 nltk 和机器学习库 scikit-learn:
```python
# 导入必要的库
import nltk
import numpy as np
import random
import string
# 读取文本文件并进行预处理
f = open('chatbot.txt','r',errors = 'ignore')
raw = f.read()
raw = raw.lower()
nltk.download('punkt')
nltk.download('wordnet')
sent_tokens = nltk.sent_tokenize(raw)
word_tokens = nltk.word_tokenize(raw)
# 定义一个函数来对用户的输入进行标准化和归一化处理
lemmer = nltk.stem.WordNetLemmatizer()
def LemTokens(tokens):
return [lemmer.lemmatize(token) for token in tokens]
remove_punct_dict = dict((ord(punct), None) for punct in string.punctuation)
def LemNormalize(text):
return LemTokens(nltk.word_tokenize(text.lower().translate(remove_punct_dict)))
# 定义一个函数来响应用户的问候
GREETING_INPUTS = ("hello", "hi", "greetings", "sup", "what's up", "hey",)
GREETING_RESPONSES = ["hi", "hey", "*nods*", "hi there", "hello", "I am glad! You are talking to me"]
def greeting(sentence):
for word in sentence.split():
if word.lower() in GREETING_INPUTS:
return random.choice(GREETING_RESPONSES)
# 定义一个函数来生成机器人的回应
from sklearn.feature_extraction.text import TfidfVectorizer
from sklearn.metrics.pairwise import cosine_similarity
def response(user_response):
robo_response=''
sent_tokens.append(user_response)
TfidfVec = TfidfVectorizer(tokenizer=LemNormalize, stop_words='english')
tfidf = TfidfVec.fit_transform(sent_tokens)
vals = cosine_similarity(tfidf[-1], tfidf)
idx=vals.argsort()[0][-2]
flat = vals.flatten()
flat.sort()
req_tfidf = flat[-2]
if(req_tfidf==0):
robo_response=robo_response+"I am sorry! I don't understand you"
return robo_response
else:
robo_response = robo_response+sent_tokens[idx]
return robo_response
# 与用户进行交互
flag=True
print("ROBO: My name is Robo. I will answer your queries about Chatbots. If you want to exit, type Bye!")
while(flag==True):
user_response = input()
user_response=user_response.lower()
if(user_response!='bye'):
if(user_response=='thanks' or user_response=='thank you' ):
flag=False
print("ROBO: You are welcome..")
else:
if(greeting(user_response)!=None):
print("ROBO: "+greeting(user_response))
else:
print("ROBO: ",end="")
print(response(user_response))
sent_tokens.remove(user_response)
else:
flag=False
print("ROBO: Bye! take care..")
```
这段代码实现了一个简单的聊天机器人,可以回答用户有关聊天机器人的问题。您可以在此基础上进行修改和扩展,以创建自己的聊天机器人。
生成一个聊天机器人代码
生成一个聊天机器人的代码需要进行以下步骤:
1. 收集语料库:聊天机器人需要一个语料库来学习对话。你可以使用开放的数据集,如Cornell电影对话语料库,或者自己创建一个语料库。
2. 进行数据预处理:对数据进行清洗和预处理,包括分词、去除停用词、词形还原等。
3. 训练模型:使用机器学习算法如递归神经网络(RNN)或Transformer来训练模型。
4. 构建聊天机器人:使用训练好的模型和一些规则来构建聊天机器人。
以下是一个简单的聊天机器人代码示例:
```python
# 导入必要的库
import random
import string
import nltk
from sklearn.feature_extraction.text import TfidfVectorizer
from sklearn.metrics.pairwise import cosine_similarity
# 收集语料库
corpus = ['Hi', 'Hello', 'How are you?', 'I am fine, thank you.', 'What is your name?', 'My name is Chatbot.', 'Goodbye', 'See you later']
# 进行数据预处理
nltk.download('punkt')
nltk.download('wordnet')
lemmer = nltk.stem.WordNetLemmatizer()
def LemTokens(tokens):
return [lemmer.lemmatize(token) for token in tokens]
remove_punct_dict = dict((ord(punct), None) for punct in string.punctuation)
def Normalize(text):
return LemTokens(nltk.word_tokenize(text.lower().translate(remove_punct_dict)))
# 训练模型
vectorizer = TfidfVectorizer(tokenizer=Normalize, stop_words='english')
X = vectorizer.fit_transform(corpus)
# 构建聊天机器人
def ChatBotResponse(user_response):
robo_response = ''
response_list = []
response_list.append(user_response)
tfidf = vectorizer.transform(response_list)
cos_sim = cosine_similarity(tfidf[-1], X)
idx = cos_sim.argsort()[0][-2]
flat = cos_sim.flatten()
flat.sort()
req_tfidf = flat[-2]
if(req_tfidf == 0):
robo_response = robo_response + "I am sorry, I do not understand."
return robo_response
else:
robo_response = robo_response + corpus[idx]
return robo_response
print("Chatbot: Hi, I'm a chatbot. How can I help you today?")
while True:
user_response = input("You: ")
user_response = user_response.lower()
if user_response != 'bye':
if user_response == 'thanks' or user_response == 'thank you':
print("Chatbot: You are welcome.")
else:
if ChatBotResponse(user_response) != "I am sorry, I do not understand.":
print("Chatbot: " + ChatBotResponse(user_response))
else:
print("Chatbot: I am sorry, I do not understand.")
else:
print("Chatbot: Goodbye! Take care.")
break
```
这个聊天机器人使用了TF-IDF向量化和余弦相似性来计算用户输入和语料库中回答之间的相似度,并返回最相关的回答。你可以根据自己的需求进行修改和扩展。
阅读全文
相关推荐
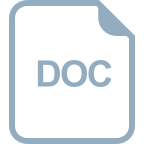
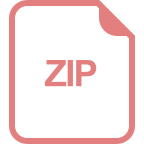
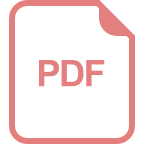
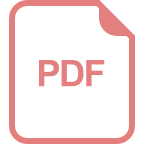
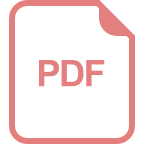
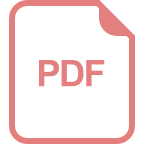

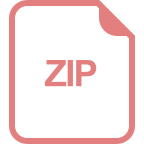
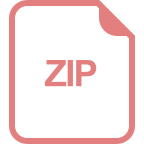
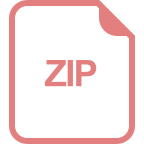
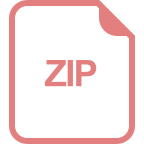
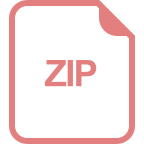