编写一个汉英小字典(Dictionary.java),将中文词作为键key、英文词作为值value所构成的“键-值”对添加到HashMap对象中,然后对其进行操作(操作随意,可排序,可遍历,同时熟悉并练习调用HashMap对象的一些常用方法)。
时间: 2023-09-10 16:07:28 浏览: 83
以下是一个简单的汉英小字典示例程序:
```java
import java.util.*;
public class Dictionary {
public static void main(String[] args) {
HashMap<String, String> dict = new HashMap<String, String>();
dict.put("苹果", "apple");
dict.put("香蕉", "banana");
dict.put("橙子", "orange");
dict.put("草莓", "strawberry");
dict.put("葡萄", "grape");
dict.put("菠萝", "pineapple");
// 遍历所有键和值
for (Map.Entry<String, String> entry : dict.entrySet()) {
System.out.println(entry.getKey() + ":" + entry.getValue());
}
// 获取某个键对应的值
String apple = dict.get("苹果");
System.out.println("苹果的英文名是:" + apple);
// 判断是否包含某个键
boolean containsKey = dict.containsKey("草莓");
System.out.println("字典中是否包含草莓:" + containsKey);
// 判断是否包含某个值
boolean containsValue = dict.containsValue("orange");
System.out.println("字典中是否包含orange:" + containsValue);
// 移除某个键值对
dict.remove("菠萝");
System.out.println("移除菠萝后的字典内容:");
for (Map.Entry<String, String> entry : dict.entrySet()) {
System.out.println(entry.getKey() + ":" + entry.getValue());
}
// 获取所有键
Set<String> keys = dict.keySet();
System.out.println("字典中的所有键:");
for (String key : keys) {
System.out.println(key);
}
// 获取所有值
Collection<String> values = dict.values();
System.out.println("字典中的所有值:");
for (String value : values) {
System.out.println(value);
}
}
}
```
以上程序创建了一个HashMap对象,然后添加了若干个键值对,最后演示了HashMap对象的几个常用方法,包括遍历所有键和值、获取某个键对应的值、判断是否包含某个键或值、移除某个键值对、获取所有键和所有值。您可以根据自己的需求修改或扩展该程序。
相关推荐
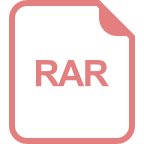









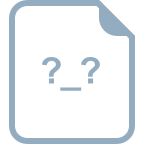