你能用java写一个hyperledger fabric平台的auction智能合约链码吗
时间: 2024-05-02 20:17:10 浏览: 28
当然可以,以下是一个简单的Hyperledger Fabric平台的auction智能合约链码的Java实现示例:
```java
package org.example.auction;
import org.hyperledger.fabric.contract.Context;
import org.hyperledger.fabric.contract.ContractInterface;
import org.hyperledger.fabric.contract.annotation.Contract;
import org.hyperledger.fabric.contract.annotation.Default;
import org.hyperledger.fabric.contract.annotation.Info;
import org.hyperledger.fabric.contract.annotation.Transaction;
import org.hyperledger.fabric.shim.ChaincodeException;
import org.hyperledger.fabric.shim.ChaincodeStub;
import org.json.JSONObject;
import java.nio.charset.StandardCharsets;
import java.util.ArrayList;
import java.util.List;
@Contract(
name = "auction",
info = @Info(
title = "Auction Contract",
description = "Auction Smart Contract",
version = "1.0"
)
)
@Default
public final class Auction implements ContractInterface {
private enum AssetStatus {
FOR_SALE,
SOLD,
REMOVED
}
private static final String ASSET_PREFIX = "asset";
private static final String OWNER_PREFIX = "owner";
private static final String BID_PREFIX = "bid";
@Transaction
public void createAsset(Context ctx, String assetId, String owner, String description, int reservePrice) {
ChaincodeStub stub = ctx.getStub();
if (assetExists(stub, assetId)) {
throw new ChaincodeException("Asset " + assetId + " already exists");
}
Asset asset = new Asset(description, owner, reservePrice, AssetStatus.FOR_SALE);
String assetJSON = asset.toJSONString();
stub.putStringState(ASSET_PREFIX + assetId, assetJSON);
stub.putStringState(OWNER_PREFIX + assetId, owner);
}
@Transaction
public String readAsset(Context ctx, String assetId) {
ChaincodeStub stub = ctx.getStub();
String assetJSON = stub.getStringState(ASSET_PREFIX + assetId);
if (assetJSON == null || assetJSON.isEmpty()) {
throw new ChaincodeException("Asset " + assetId + " does not exist");
}
return assetJSON;
}
@Transaction
public void updateAsset(Context ctx, String assetId, String newOwner, String newDescription, int newReservePrice) {
ChaincodeStub stub = ctx.getStub();
if (!assetExists(stub, assetId)) {
throw new ChaincodeException("Asset " + assetId + " does not exist");
}
Asset asset = new Asset(newDescription, newOwner, newReservePrice, AssetStatus.FOR_SALE);
String assetJSON = asset.toJSONString();
stub.putStringState(ASSET_PREFIX + assetId, assetJSON);
stub.putStringState(OWNER_PREFIX + assetId, newOwner);
}
@Transaction
public void removeAsset(Context ctx, String assetId) {
ChaincodeStub stub = ctx.getStub();
if (!assetExists(stub, assetId)) {
throw new ChaincodeException("Asset " + assetId + " does not exist");
}
stub.delState(ASSET_PREFIX + assetId);
stub.delState(OWNER_PREFIX + assetId);
List<Bid> bids = getAllBidsForAsset(ctx, assetId);
for (Bid bid : bids) {
stub.delState(BID_PREFIX + bid.getId());
}
Asset asset = new Asset("", "", 0, AssetStatus.REMOVED);
String assetJSON = asset.toJSONString();
stub.putStringState(ASSET_PREFIX + assetId, assetJSON);
}
@Transaction
public void placeBid(Context ctx, String assetId, String bidder, int amount) {
ChaincodeStub stub = ctx.getStub();
if (!assetExists(stub, assetId)) {
throw new ChaincodeException("Asset " + assetId + " does not exist");
}
if (!isAssetForSale(stub, assetId)) {
throw new ChaincodeException("Asset " + assetId + " is not for sale");
}
List<Bid> bids = getAllBidsForAsset(ctx, assetId);
int highestBid = getHighestBidAmount(bids);
if (highestBid >= amount) {
throw new ChaincodeException("Bid amount must be higher than the current highest bid");
}
Bid bid = new Bid(assetId, bidder, amount);
String bidJSON = bid.toJSONString();
stub.putStringState(BID_PREFIX + bid.getId(), bidJSON);
}
@Transaction
public String getHighestBid(Context ctx, String assetId) {
ChaincodeStub stub = ctx.getStub();
List<Bid> bids = getAllBidsForAsset(ctx, assetId);
int highestBid = getHighestBidAmount(bids);
JSONObject result = new JSONObject();
result.put("asset_id", assetId);
result.put("highest_bid", highestBid);
return result.toString();
}
private boolean assetExists(ChaincodeStub stub, String assetId) {
String assetJSON = stub.getStringState(ASSET_PREFIX + assetId);
return assetJSON != null && !assetJSON.isEmpty();
}
private boolean isAssetForSale(ChaincodeStub stub, String assetId) {
String assetJSON = stub.getStringState(ASSET_PREFIX + assetId);
if (assetJSON == null || assetJSON.isEmpty()) {
return false;
}
Asset asset = Asset.fromJSONString(assetJSON);
return asset.getStatus() == AssetStatus.FOR_SALE;
}
private List<Bid> getAllBidsForAsset(Context ctx, String assetId) {
ChaincodeStub stub = ctx.getStub();
List<Bid> bids = new ArrayList<>();
String startKey = BID_PREFIX + "0";
String endKey = BID_PREFIX + "zzz";
List<String> bidKeys = stub.getStateByRange(startKey, endKey);
for (String bidKey : bidKeys) {
String bidJSON = stub.getStringState(bidKey);
Bid bid = Bid.fromJSONString(bidJSON);
if (bid.getAssetId().equals(assetId)) {
bids.add(bid);
}
}
return bids;
}
private int getHighestBidAmount(List<Bid> bids) {
int highestBid = 0;
for (Bid bid : bids) {
if (bid.getAmount() > highestBid) {
highestBid = bid.getAmount();
}
}
return highestBid;
}
private static final class Asset {
private final String description;
private final String owner;
private final int reservePrice;
private final AssetStatus status;
Asset(String description, String owner, int reservePrice, AssetStatus status) {
this.description = description;
this.owner = owner;
this.reservePrice = reservePrice;
this.status = status;
}
static Asset fromJSONString(String assetJSON) {
JSONObject obj = new JSONObject(assetJSON);
String description = obj.getString("description");
String owner = obj.getString("owner");
int reservePrice = obj.getInt("reserve_price");
AssetStatus status = AssetStatus.valueOf(obj.getString("status"));
return new Asset(description, owner, reservePrice, status);
}
String toJSONString() {
JSONObject obj = new JSONObject();
obj.put("description", description);
obj.put("owner", owner);
obj.put("reserve_price", reservePrice);
obj.put("status", status.toString());
return obj.toString();
}
AssetStatus getStatus() {
return status;
}
}
private static final class Bid {
private final String id;
private final String assetId;
private final String bidder;
private final int amount;
Bid(String assetId, String bidder, int amount) {
this.id = assetId + "_" + bidder;
this.assetId = assetId;
this.bidder = bidder;
this.amount = amount;
}
String toJSONString() {
JSONObject obj = new JSONObject();
obj.put("id", id);
obj.put("asset_id", assetId);
obj.put("bidder", bidder);
obj.put("amount", amount);
return obj.toString();
}
String getId() {
return id;
}
String getAssetId() {
return assetId;
}
int getAmount() {
return amount;
}
static Bid fromJSONString(String bidJSON) {
JSONObject obj = new JSONObject(bidJSON);
String id = obj.getString("id");
String assetId = obj.getString("asset_id");
String bidder = obj.getString("bidder");
int amount = obj.getInt("amount");
Bid bid = new Bid(assetId, bidder, amount);
bid.id = id;
return bid;
}
}
}
```
这个智能合约链码实现了创建,读取,更新和删除资产以及竞标功能。它使用JSON格式存储资产和竞标,并使用链码存储和检索这些数据。这只是一个简单的实现示例,实际应用中可能需要更多的功能和安全性。
相关推荐
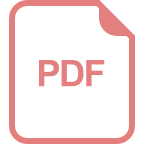
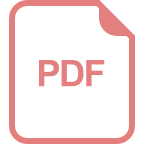
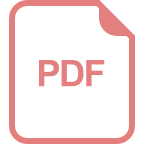














