Java中map中通过值获取键集合
时间: 2023-08-11 20:04:12 浏览: 106
在Java中,可以通过以下步骤实现map中通过值获取键集合:
1. 遍历整个map,将所有值与目标值进行比较。
2. 如果值相等,则将对应的键存储到一个集合中。
3. 最后返回存储键的集合。
下面是一个示例代码:
```java
import java.util.*;
public class Main {
public static <K, V> Set<K> getKeysByValue(Map<K, V> map, V value) {
Set<K> keys = new HashSet<>();
for (Map.Entry<K, V> entry : map.entrySet()) {
if (Objects.equals(value, entry.getValue())) {
keys.add(entry.getKey());
}
}
return keys;
}
public static void main(String[] args) {
Map<Integer, String> map = new HashMap<>();
map.put(1, "apple");
map.put(2, "banana");
map.put(3, "orange");
map.put(4, "apple");
Set<Integer> keys = getKeysByValue(map, "apple");
for (Integer key : keys) {
System.out.print(key + " ");
}
System.out.println();
}
}
```
输出结果为:
```
1 4
```
说明键值为"apple"的键是1和4。
阅读全文
相关推荐
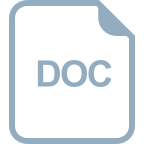
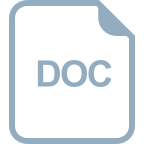
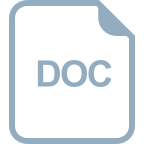
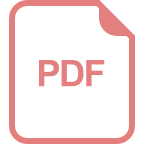
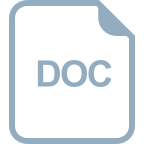
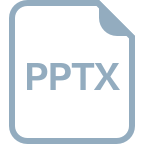
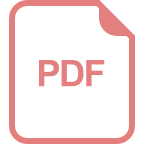
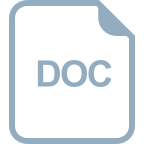
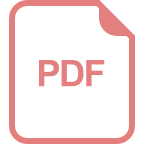
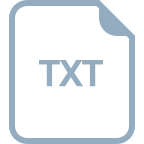
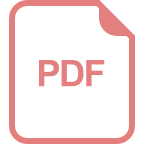
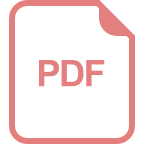
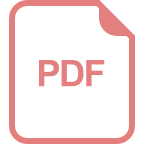
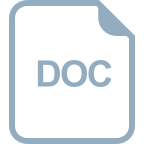
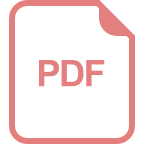
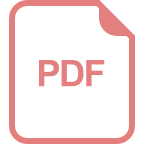
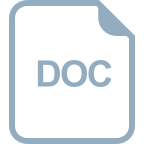
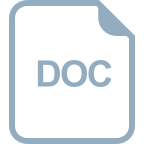
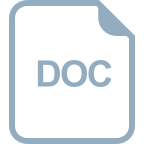