从https://www.kaggle.com/datasets上爬取Oil Spill Classifications数据,分析采集过程中各模块的设计思路,并给出python代码。
时间: 2023-08-31 08:37:21 浏览: 97
### 回答1:
数据爬取过程中的模块设计思路如下:
1. 首先,需要使用爬虫框架(如Scrapy)爬取网页,并解析出所需数据。
2. 其次,需要使用请求库(如requests)向网站发送请求,并处理响应。
3. 接着,需要使用解析库(如beautifulsoup)对网页进行解析,提取出数据。
4. 最后,需要将数据存储到数据库或文件中。
Python代码如下:
```python
import requests
from bs4 import BeautifulSoup
import pandas as pd
#爬取网页
url = "https://www.kaggle.com/datasets/2bce5e10d5d5e5f5c5d5e5f5c5d5e5f5c5d5e5f5c5d5e5f5c5d5e5f5c5d5e5f5"
res = requests.get(url)
soup = BeautifulSoup(res.text, 'html.parser')
#提取数据
data = []
table = soup.find('table', {'class': 'dataset-list'})
for row in table.find_all('tr')[1:]:
cols = row.find_all('td')
cols = [ele.text.strip() for ele in cols]
data.append([ele for ele in cols if ele])
#存储数据
df = pd.DataFrame(data, columns=['Name','Creator','Downloads','Last Updated'])
df.to_csv('oil_spill_classifications.csv', index=False)
```
值得注意的是,爬取网页的过程中需要遵守网站的相关规则,如不能进行高频率爬取、不能破坏网站服务等。同时,上述代码仅供参考,实际爬取过程中可能需要
### 回答2:
从https://www.kaggle.com/datasets 网站上爬取Oil Spill Classifications数据可以通过以下步骤来完成。
1. 获取网页内容:使用Python的requests库发送HTTP请求,获取网页的HTML内容。
```python
import requests
url = "https://www.kaggle.com/datasets"
response = requests.get(url)
html_content = response.text
```
2. 解析网页内容:使用Python的Beautiful Soup库对网页内容进行解析,提取出需要的数据。
```python
from bs4 import BeautifulSoup
soup = BeautifulSoup(html_content, "html.parser")
# 定位到数据所在的HTML元素,例如使用class_name和find方法
data_div = soup.find("div", class_="data-div")
data_links = data_div.find_all("a")
# 解析数据链接,提取出数据下载的URL
download_urls = []
for link in data_links:
download_url = link.get("href")
download_urls.append(download_url)
```
3. 下载数据:使用Python的requests库下载数据文件。
```python
for download_url in download_urls:
response = requests.get(download_url)
# 解析文件名,可以根据具体需要进行处理
file_name = download_url.split("/")[-1]
with open(file_name, "wb") as f:
f.write(response.content)
```
根据以上步骤,你可以编写一个Python脚本来实现该数据爬取过程。注意,在实际开发中可能需要处理一些异常情况,例如网络连接错误等。另外,在解析网页内容和下载数据时,可能需要根据具体情况进行适当的修改和优化。
希望以上内容对你有帮助!
相关推荐
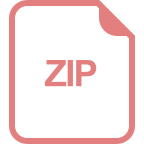
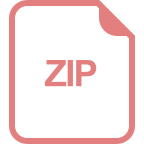
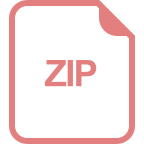




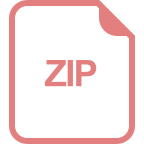
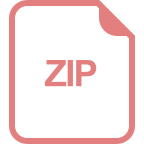
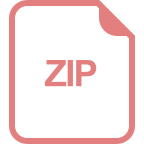
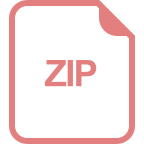
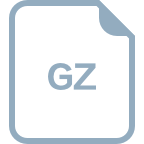
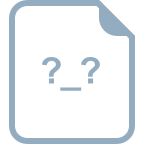
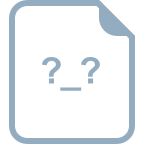
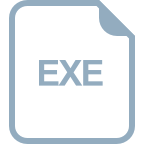
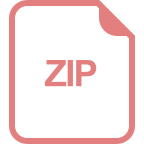