在unity中使用C#实现 在一个位置生成5个金币然后每隔0.2秒依次飞向指定位置 最后到达该位置后销毁
时间: 2024-03-17 09:39:57 浏览: 117
可以使用 Unity 的协程(Coroutine)来实现这个需求。以下是一个简单的实现:
```csharp
using UnityEngine;
using System.Collections;
public class CoinGenerator : MonoBehaviour
{
public GameObject coinPrefab;
public Transform target;
public float delay = 0.2f;
public float speed = 5f;
void Start()
{
StartCoroutine(SpawnCoins());
}
IEnumerator SpawnCoins()
{
for (int i = 0; i < 5; i++)
{
GameObject coin = Instantiate(coinPrefab, transform.position, Quaternion.identity);
StartCoroutine(MoveCoin(coin, i));
yield return new WaitForSeconds(delay);
}
}
IEnumerator MoveCoin(GameObject coin, int index)
{
Vector3 startPos = coin.transform.position;
Vector3 endPos = target.position;
endPos.y = startPos.y;
float t = 0f;
while (t < 1f)
{
t += Time.deltaTime * speed;
coin.transform.position = Vector3.Lerp(startPos, endPos, t);
yield return null;
}
if (index == 4)
{
Destroy(gameObject);
}
else
{
Destroy(coin);
}
}
}
```
在这个脚本中,我们使用 `StartCoroutine()` 方法来启动协程。`SpawnCoins()` 协程会生成 5 个金币,并在每个金币生成后等待 0.2 秒。每个金币通过 `MoveCoin()` 协程来移动,移动过程中使用 `Lerp()` 方法实现平滑移动。当最后一个金币到达目标位置后,销毁当前物体;否则,销毁当前金币。
需要注意的是,我们需要将金币的 Y 坐标与起始位置的 Y 坐标保持一致,以避免金币在垂直方向上飞行。另外,我们使用 `Destroy()` 方法来销毁物体,这可以确保对象在协程结束后被正确地销毁。
阅读全文
相关推荐
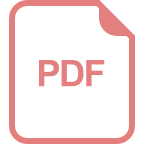
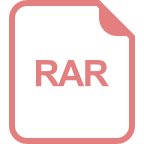
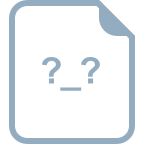
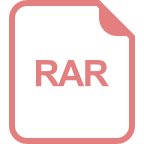
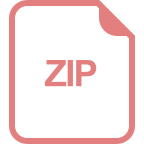
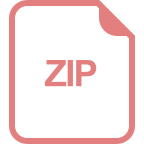
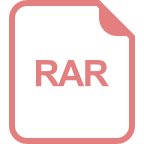
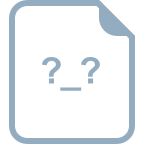
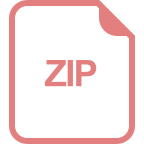
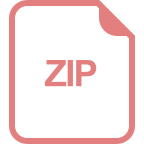
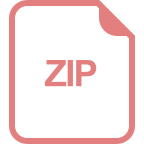
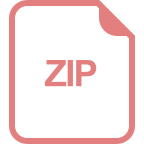
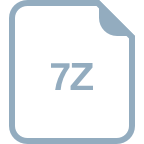
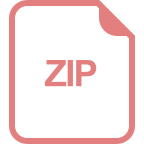
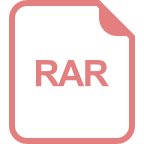