csharp的DataSource是list,怎样将DataSource转换回来
时间: 2023-11-23 09:07:29 浏览: 24
可以使用ToList()方法将DataSource转换为List。示例代码如下:
List<MyObject> list = dataGridView.DataSource as List<MyObject>;
if (list != null)
{
// TODO: 对List进行操作
}
相关问题
// 将List绑定到List控件 listBox.DataSource = dataList;报错
如果您在将List绑定到List控件时遇到了错误,可能是因为List控件无法直接将List作为数据源。您可以尝试使用BindingSource作为中间层来实现数据绑定。以下是修改后的代码示例:
```csharp
using System;
using System.Collections.Generic;
using System.Data;
using System.Data.SqlClient;
using System.Windows.Forms;
namespace ListExample
{
public partial class MainForm : Form
{
private string connectionString = "YourConnectionString"; // 替换为您的数据库连接字符串
public MainForm()
{
InitializeComponent();
}
private void MainForm_Load(object sender, EventArgs e)
{
LoadData();
}
private void LoadData()
{
// 创建连接对象
using (SqlConnection connection = new SqlConnection(connectionString))
{
// 创建查询语句
string query = "SELECT * FROM YourTableName"; // 替换为您的表名
// 打开数据库连接
connection.Open();
// 创建Command对象
SqlCommand command = new SqlCommand(query, connection);
// 执行查询并获取DataReader对象
SqlDataReader reader = command.ExecuteReader();
// 创建一个List来存储数据
List<string> dataList = new List<string>();
// 读取数据并添加到List中
while (reader.Read())
{
string data = reader["ColumnName"].ToString(); // 替换为您的列名
dataList.Add(data);
}
// 关闭DataReader
reader.Close();
// 创建BindingSource对象,并将List作为数据源
BindingSource bindingSource = new BindingSource();
bindingSource.DataSource = dataList;
// 将BindingSource绑定到List控件
listBox.DataSource = bindingSource;
}
}
}
}
```
在这个示例中,我们使用了BindingSource作为中间层来将List作为数据源绑定到List控件。这样就可以解决直接将List绑定到List控件时的错误。如果问题仍然存在,请提供具体的错误信息,以便我能够更好地帮助您解决问题。
DataGridView1.DataSource转成list对象
可以通过以下代码将 DataGridView1 的 DataSource 转换为 List 对象:
```csharp
List<YourClass> list = new List<YourClass>();
foreach (DataGridViewRow row in dataGridView1.Rows)
{
YourClass obj = new YourClass();
obj.Property1 = row.Cells["Column1"].Value.ToString();
obj.Property2 = row.Cells["Column2"].Value.ToString();
// 将其他属性赋值给 obj
list.Add(obj);
}
```
其中,YourClass 是你自己定义的类,用于存储 DataGridView 中的数据。在 foreach 循环中,依次遍历 DataGridView 中的每一行,将每一行的数据赋值给 YourClass 对象,并将对象添加到 List 中。这样就可以将 DataGridView 的数据转换为 List 对象了。
相关推荐
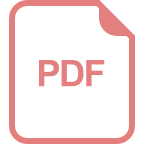
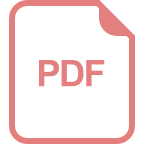
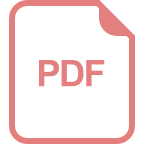












