mybatisplus apply in
时间: 2024-06-14 08:02:42 浏览: 8
MyBatis-Plus是一个MyBatis的增强工具,在MyBatis的基础上进行了扩展和增强,简化了开发流程,提高了开发效率。其中,apply是MyBatis-Plus提供的一种高级查询方法,可以用于实现复杂的查询操作。
apply方法可以通过SQL子查询来实现多表关联查询,也可以使用内置的函数实现一些复杂的计算和筛选操作。在使用apply方法时,需要在Mapper接口中定义一个返回值为IPage<T>类型的方法,并在方法上添加@Select注解,然后在注解中编写SQL语句。
以下是一个使用apply方法实现多表关联查询的示例:
```
@Select("select t.*, o.order_no from t_user t " +
"left join (select * from t_order) o " +
"on t.id = o.user_id " +
"where t.id = #{userId}")
IPage<UserOrderVO> selectUserOrder(Page<UserOrderVO> page, @Param("userId") Long userId);
```
以上示例中,使用了SQL子查询来实现了t_user表和t_order表的关联查询,并且在查询结果中加入了订单号order_no字段。同时,使用了MyBatis-Plus提供的分页功能,将查询结果封装到了IPage<UserOrderVO>类型中。
相关问题
mybatisplus apply
MyBatis Plus is a powerful extension library for MyBatis, a popular Java-based persistence framework. It provides additional features and utilities to simplify and enhance the usage of MyBatis. One of its core features is the "apply" method, which allows you to dynamically apply query conditions to a SQL statement.
The "apply" method in MyBatis Plus is typically used in combination with the "Wrapper" interface. The "Wrapper" interface provides a set of methods for building query conditions, such as "eq" (equal), "like", "in", etc. By using the "apply" method, you can dynamically apply these query conditions to your SQL statement based on certain conditions.
Here's an example of how to use the "apply" method in MyBatis Plus:
```java
import com.baomidou.mybatisplus.core.conditions.query.QueryWrapper;
import com.baomidou.mybatisplus.core.toolkit.Wrappers;
public class MyBatisPlusExample {
public List<User> getUsers(String name, Integer age) {
QueryWrapper<User> queryWrapper = Wrappers.query();
queryWrapper.eq("status", 1)
.like(StringUtils.isNotBlank(name), "name", name)
.lt(age != null, "age", age);
// Apply the query conditions to the SQL statement
queryWrapper.apply("date_format(create_time, '%Y-%m-%d') = {0}", "2022-01-01");
return userDao.selectList(queryWrapper);
}
}
```
In the above example, we create a `QueryWrapper` object and use various methods to build our query conditions. We then use the `apply` method to dynamically apply the additional condition `date_format(create_time, '%Y-%m-%d') = '2022-01-01'` to the SQL statement.
By using the `apply` method, you can easily add custom conditions to your SQL statements based on dynamic requirements. This can be particularly useful when you need to apply complex conditions that cannot be easily expressed using the provided query methods in MyBatis Plus.
mybatisplus find_in_set
MyBatis Plus doesn't have a built-in function for `FIND_IN_SET` like MySQL does. However, you can achieve similar functionality using the `Sqls` class provided by MyBatis Plus.
Here's an example of how you can use the `Sqls` class to simulate the `FIND_IN_SET` function in MyBatis Plus:
```java
import com.baomidou.mybatisplus.core.conditions.query.QueryWrapper;
import com.baomidou.mybatisplus.core.toolkit.sql.SqlUtils;
...
QueryWrapper<Entity> queryWrapper = new QueryWrapper<>();
queryWrapper.apply(SqlUtils.format("FIND_IN_SET({0}, column_name) > 0", value));
List<Entity> result = entityMapper.selectList(queryWrapper);
```
In the above example, `column_name` is the name of the column you want to search in, and `value` is the value you want to find within the set.
The `SqlUtils.format` method helps format the SQL statement with the desired parameters.
Please note that this approach may not be as efficient as using the native `FIND_IN_SET` function in MySQL, as it requires additional processing in Java.
相关推荐
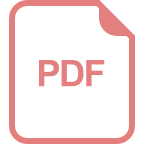












