candidate function not viable: no known conversion from 'int' to 'int &&' for 1st argument
时间: 2024-10-17 14:10:43 浏览: 25
这个错误信息出现在C++中,表示你在试图通过隐式或强制类型转换将一个整型变量(`int`)转换成引用(`int&&`)时遇到了问题。在C++11及以后的标准中,`int &&`通常用于捕获移动构造或赋值运算中的左操作数,它实际上是一种特殊的右值引用。
错误消息中的“candidate function not viable”意味着编译器找到了一个候选函数,但它不适合当前的场景。在这个例子中,函数的第1个参数应该接收一个右值引用,但你提供的却是普通整数值,所以编译器找不到合适的转换路径。
例如,下面的代码会导致这种错误:
```cpp
void takeIntByMove(int &&value) {
// 函数预期一个右值引用
}
int main() {
int x = 5;
takeIntByMove(x); // 错误,x不是一个右值引用
}
```
为了修复这个错误,你需要确保传递给`takeIntByMove`的是一个右值。如果是从函数内部返回,你可以直接返回一个右值,如果是外部的,你可以考虑使用`move()`函数将其转换为右值引用:
```cpp
int main() {
int x = 5;
takeIntByMove(move(x)); // 现在x被移到并传入函数,正确
}
```
相关问题
monitorsystem.cpp:292:5: error: no matching function for call to 'number' qstring.h:757:20: note: candidate function not viable: no known conversion from 'quint8 [6]' to 'int' for 1st argument qstring.h:758:20: note: candidate function not viable: no known conversion from 'quint8 [6]' to 'uint' (aka 'unsigned int') for 1st argument qstring.h:759:20: note: candidate function not viable: no known conversion from 'quint8 [6]' to 'long' for 1st argument qstring.h:760:20: note: candidate function not viable: no known conversion from 'quint8 [6]' to 'ulong' (aka 'unsigned long') for 1st argument qstring.h:761:20: note: candidate function not viable: no known conversion from 'quint8 [6]' to 'qlonglong' (aka 'long long') for 1st argument qstring.h:762:20: note: candidate function not viable: no known conversion from 'quint8 [6]' to 'qulonglong' (aka 'unsigned long long') for 1st argument qstring.h:763:20: note: candidate function not viable: no known conversion from 'quint8 [6]' to 'double' for 1st argument
这个错误提示是因为在调用 QString::number() 函数时,将一个 quint8 数组作为参数传递给了该函数,但是 QString::number() 函数没有接受 quint8 数组作为参数的重载函数。
如果要将 quint8 数组转换为 QString 类型,可以使用 QByteArray 类型的 fromRawData() 函数和 QString 的构造函数。具体做法如下:
```
quint8 a[6] = {1, 2, 3, 4, 5, 6};
int size = sizeof(quint8) * 6;
QString result = QString::fromUtf8(QByteArray::fromRawData(reinterpret_cast<const char*>(a), size), size);
// result 的值为 ""
```
在这个例子中,fromRawData() 函数接受两个参数:一个指向字符数组的指针和数组的大小。首先将 quint8 数组转换为 char 数组的指针,然后使用该指针和数组的大小作为参数调用 fromRawData() 函数,得到一个 QByteArray 类型的对象。接着使用 QString 的构造函数将 QByteArray 类型的对象转换为 QString 类型的字符串。需要注意的是,构造函数的第二个参数指定的是字符串的长度。在这个例子中,由于 quint8 数组的大小为 6,因此字符串的长度也应该为 6。
http_request.cpp:177:21: No matching function for call to 'information' qmessagebox.h:217:34: candidate function not viable: no known conversion from 'Http_request *' to 'QWidget *' for 1st argument qmessagebox.h:207:16: candidate function not viable: no known conversion from 'Http_request *' to 'QWidget *' for 1st argument qmessagebox.h:185:27: candidate function not viable: no known conversion from 'Http_request *' to 'QWidget *' for 1st argument qmessagebox.h:210:16: candidate function not viable: no known conversion from 'Http_request *' to 'QWidget *' for 1st argument
根据错误提示,你正在从`Http_request`类中调用QMessageBox::information()函数,但是该函数需要一个QWidget类型的参数作为父窗口,而`Http_request`不是QWidget的子类。因此,你需要传递一个有效的QWidget对象作为父窗口,例如:
```
QMessageBox::information(this, "提示", "操作成功!", QMessageBox::Yes);
```
其中,this指针代表当前的QWidget对象,可以作为父窗口传递给QMessageBox::information()函数。如果你在其他的类中使用QMessageBox::information()函数,需要传递一个有效的QWidget对象作为父窗口。
阅读全文
相关推荐
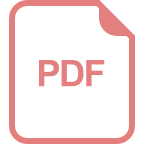
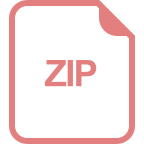
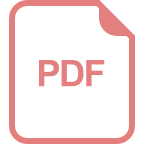














