swt页面中如何修改数据库中的表的具体代码
时间: 2024-02-04 22:04:25 浏览: 26
在 SWT 页面中修改数据库中表的具体代码需要通过以下几个步骤:
1. 连接数据库:使用 JDBC 连接数据库,获取数据库连接对象 Connection。
2. 准备 SQL 语句:根据需要修改的表的具体代码编写 SQL 语句,如 ALTER TABLE 语句等。
3. 执行 SQL 语句:通过 Connection 对象创建 Statement 或 PreparedStatement 对象,并执行 SQL 语句。
4. 处理结果:根据需要处理执行 SQL 语句后返回的结果,如修改的行数等。
下面是一个简单的示例代码:
```java
// 获取数据库连接
Connection conn = DriverManager.getConnection("jdbc:mysql://localhost:3306/test", "root", "123456");
// 准备 SQL 语句
String sql = "ALTER TABLE users ADD COLUMN age INT(3)";
// 创建 Statement 对象
Statement stmt = conn.createStatement();
// 执行 SQL 语句
int rows = stmt.executeUpdate(sql);
// 处理结果
System.out.println("修改了 " + rows + " 行");
```
需要注意的是,上述代码仅供参考,具体实现可能需要根据具体情况进行调整。同时,修改数据库表结构需要谨慎操作,务必备份数据并进行充分测试。
相关问题
swt页面中如何修改数据库中的表的具体实行代码
1;
const far = 100;
matrix.perspective(fovy, aspect, near, far);
return matrix;
}
//在 SWT 页面中修改数据库中表的具体实行代码需要在 SWT 的事件处理器中编写相应的代码。 矩阵相乘
function multiplyMatrix(matrix1, matrix2) {
const matrix = identityMatrix();
for (let i = 具体实现可以参考以下步骤:
1. 在 SWT 页面中添加相应的控件,如按钮,文本0; i < 4; i++) {
for (let j = 0; j < 4; j++) {
let框等。
2. 在按钮的 SelectionListener 中编写事件处理器,用于连接数据库并执行 SQL 语句。
``` sum = 0;
for (let k = 0; k < 4; k++) {
sum += matrix1.get(ijava
button.addSelectionListener(new SelectionAdapter() {
@Override
public void widgetSelected(SelectionEvent e) {
try {
, k) * matrix2.get(k, j);
}
matrix.set(i, j, sum);
}
}
return matrix;
}
// 创建单位矩阵
function identityMatrix() {
return new Matrix([
[1, 0, 0, // 连接数据库
Connection conn = DriverManager.getConnection("jdbc:mysql://localhost:3306/test", "root", "1234560],
[0, 1, 0, 0],
[0, 0, 1, 0],
");
// 准备 SQL 语句
String sql = "ALTER TABLE users ADD COLUMN age INT(3)";
// 创建 Statement [0, 0, 0, 1]
]);
}
// 矩阵类
class Matrix {
constructor(data) {
对象
Statement stmt = conn.createStatement();
// 执行 SQL 语句
int rows = stmt.executeUpdate(sql);
// this.data = data;
}
get(row, column) {
return this.data[row][column];
}
set(row, column 处理结果,更新 UI
Display.getDefault().asyncExec(() -> {
// 更新 UI 上的文本框或标签
, value) {
this.data[row][column] = value;
}
translate(x, y, z) {
const matrix = label.setText("修改了 " + rows + " 行");
});
// 关闭连接
stmt.close();
conn.close new Matrix([
[1, 0, 0, x],
[0, 1, 0, y],
[();
} catch (SQLException ex) {
ex.printStackTrace();
}
}
});
```
需要注意的是,由于事件处理0, 0, 1, z],
[0, 0, 0, 1]
]);
this.data =器在 SWT 线程中执行,因此需要使用 Display.getDefault().asyncExec() 来更新 UI 上的控件。另外, multiplyMatrix(matrix, this).data;
}
rotateX(angle) {
const sin = Math.sin(angle);
const cos = Math为了避免阻塞 SWT 线程,建议在事件处理器中使用单独的线程来执行 SQL 语句。
swt页面中如何查询数据库中的表的具体代码
要在 SWT 页面中查询数据库中的表,需要进行以下步骤:
1. 首先,需要连接到数据库。这可以使用 JDBC (Java Database Connectivity) API 来完成。以下是连接到数据库的示例代码:
```
// 导入 JDBC API
import java.sql.*;
// 连接到数据库
Connection conn = DriverManager.getConnection("jdbc:mysql://localhost:3306/mydatabase", "username", "password");
```
2. 接下来,需要创建一个 SWT 表格并为其添加列。以下是创建 SWT 表格并添加列的示例代码:
```
// 导入 SWT API
import org.eclipse.swt.SWT;
import org.eclipse.swt.layout.FillLayout;
import org.eclipse.swt.widgets.Display;
import org.eclipse.swt.widgets.Shell;
import org.eclipse.swt.widgets.Table;
import org.eclipse.swt.widgets.TableColumn;
import org.eclipse.swt.widgets.TableItem;
// 创建 SWT 表格
Display display = new Display();
Shell shell = new Shell(display);
shell.setLayout(new FillLayout());
Table table = new Table(shell, SWT.BORDER | SWT.FULL_SELECTION);
// 添加列
TableColumn column1 = new TableColumn(table, SWT.NONE);
column1.setText("Column 1");
TableColumn column2 = new TableColumn(table, SWT.NONE);
column2.setText("Column 2");
```
3. 然后,需要使用 SQL 查询语句从数据库中检索数据。以下是使用 SQL 查询语句从数据库中检索数据的示例代码:
```
// 使用 SQL 查询语句检索数据
Statement stmt = conn.createStatement();
ResultSet rs = stmt.executeQuery("SELECT * FROM mytable");
```
4. 最后,需要将从数据库中检索到的数据添加到 SWT 表格中。以下是将数据添加到 SWT 表格中的示例代码:
```
// 将数据添加到 SWT 表格中
while (rs.next()) {
TableItem item = new TableItem(table, SWT.NONE);
item.setText(new String[] { rs.getString("column1"), rs.getString("column2") });
}
```
将以上四段代码结合在一起,即可在 SWT 页面中查询数据库中的表。如果需要实现更复杂的查询功能,可以在 SQL 查询语句中使用 WHERE 子句和其他 SQL 语句。
相关推荐
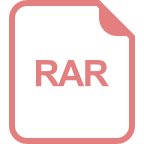
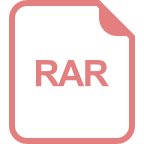
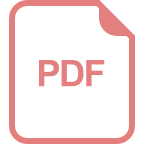












