tensorflow搭建一维DenseNet
时间: 2023-10-05 08:13:59 浏览: 107
一维的DenseNet是将多个1D卷积层和密集连接层(Dense Layer)串联起来,形成一个深层的神经网络。下面是一个使用TensorFlow搭建一维DenseNet的示例代码:
```python
import tensorflow as tf
def dense_block(x, num_layers, growth_rate, name):
with tf.variable_scope(name):
layers_concat = [x]
for i in range(num_layers):
x = tf.layers.conv1d(layers_concat[-1], growth_rate, kernel_size=3, padding='same', activation=tf.nn.relu, name='conv1d_{}'.format(i+1))
layers_concat.append(x)
x = tf.concat(layers_concat, axis=-1)
return x
def transition_layer(x, name):
with tf.variable_scope(name):
x = tf.layers.conv1d(x, int(x.get_shape()[-1]), kernel_size=1, padding='same', activation=tf.nn.relu, name='conv1d')
x = tf.layers.average_pooling1d(x, pool_size=2, strides=2, name='avg_pool')
return x
def dense_net(x, num_blocks, num_layers, growth_rate, num_classes):
# input layer
conv1 = tf.layers.conv1d(x, 2*growth_rate, kernel_size=7, padding='same', activation=tf.nn.relu, name='conv1')
# dense blocks
for i in range(num_blocks):
block = dense_block(conv1, num_layers, growth_rate, name='dense_block_{}'.format(i+1))
conv1 = tf.concat([conv1, block], axis=-1)
if i < num_blocks-1:
conv1 = transition_layer(conv1, name='transition_layer_{}'.format(i+1))
# output layer
output = tf.layers.dense(conv1, num_classes, name='output')
return output
```
以上代码的输入是一个1D的数据(比如一个时间序列),输出是这个数据所属的类别。其中,`dense_block`函数定义了一个密集块,包含多个1D卷积层和ReLU激活函数,最后将输出拼接到一起;`transition_layer`函数定义了一个过度层,包含一个1D卷积层和一个平均池化层;`dense_net`函数定义了整个DenseNet,包含多个密集块和过度层,最后输出一个向量,需要通过softmax激活函数转换为概率值。可以根据具体的需求来修改上述代码。
阅读全文
相关推荐
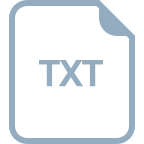
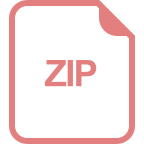
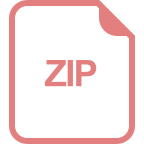
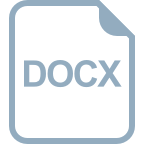
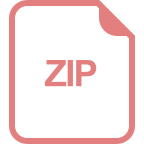
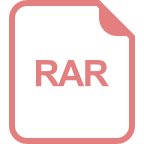
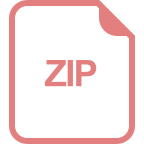
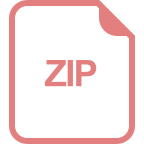
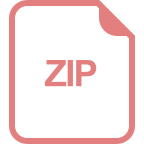
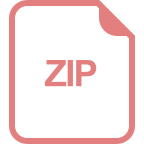
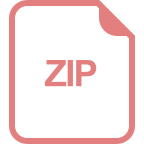
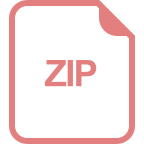
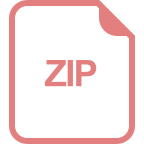
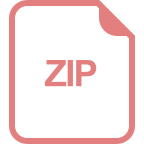