有n个学生成绩存放在数组scores中,编写写函数max,用来求学生的最高成绩;编写main函数,定义成绩数组,调用max函数,输出学生的最高成绩
时间: 2024-10-10 07:11:01 浏览: 21
在C++或者类似的语言中,可以这样设计一个简单的程序:
```cpp
#include <iostream>
// 定义函数 maxScore 来获取数组中最高的成绩
int max(int scores[], int n) {
if (n == 0) // 检查数组是否为空
return INT_MIN; // 返回最小整数防止错误
int max_score = scores[0]; // 假设第一个元素是最高的
for (int i = 1; i < n; i++) { // 遍历剩余的元素
if (scores[i] > max_score)
max_score = scores[i];
}
return max_score;
}
int main() {
// 假设我们有5个学生的成绩
int scores[] = {85, 92, 76, 98, 88};
int n = sizeof(scores) / sizeof(scores[0]); // 计算数组长度
// 调用 max 函数找到最高成绩
int highest_score = max(scores, n);
// 输出最高成绩
std::cout << "学生中的最高成绩是: " << highest_score << std::endl;
return 0;
}
```
在这个程序中,`max`函数接收一个整数数组和其长度,通过迭代找到并返回最大的成绩。在`main`函数里,我们定义了一个学生的成绩数组,并调用`max`函数来获取最高分,最后将结果打印出来。
阅读全文
相关推荐
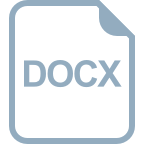
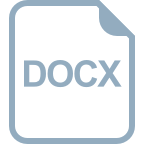
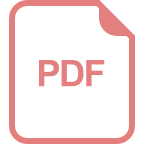













