#include<iostream> using namespace std; void showflags(long f) { long i = 0x8000; for (; i; i = i >> 1) { if (i & f) cout << "1"; else cout << "0"; } cout << endl; } int main() { showflags(cout.flags()); cout << "x_width=" << cout.width() << endl; cout << "x_fill=" << cout.fill() << endl; cout << "x_precision=" << cout.precision() << endl; cout << 123 << " " << 123.45678 << "\n"; cout << "-------------" << endl; cout << "* * * x_width=10,x_fill= ,x_precision=4 * * *" << endl<<endl<<endl<<endl; cout.width(10); cout.precision(4); cout << 123 << " " << 123.45678 << " " << 234.567 << endl;//1 cout << "x_width=" << cout.width() << endl; cout << "x_fill=" << cout.fill() << endl; cout << "x_precision=" << cout.precision() << endl; cout << "-------------" << endl; cout << "* * * x_width=10,x_fill=&,x_precision=4 * * *" << endl; cout.fill('&'); cout.width(10); cout << 123 << " " << 123.45678 << endl; cout.setf(ios::left); cout.width(10); cout << 123 << " " << 123.45678 << endl; cout << "x_width=" << cout.width() << endl; cout << "x_fill=" << cout.fill() << endl; cout << "x_precision=" << cout.precision() << endl<<endl<<endl; showflags(cout.flags());//2 cout.setf(ios::right | ios::unitbuf); cout << endl << endl << endl; showflags(cout.flags());//3 }
时间: 2023-08-20 13:11:47 浏览: 208
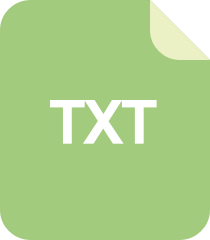
#include_iostream.h_

这段代码主要演示了 C++ 中的格式化输出,包括了流对象的标志位、宽度、填充字符和精度等属性。代码的执行流程如下:
1. 首先输出流对象 cout 的标志位,即输出格式的相关属性,使用 showflags 函数实现。
2. 输出数值 123 和 123.45678,观察宽度、填充字符和精度的默认值。
3. 更改宽度为 10,精度为 4,再次输出数值 123、123.45678 和 234.567,观察结果。
4. 更改填充字符为 &,并设置左对齐,重新输出数值 123 和 123.45678,观察结果。
具体的代码解释如下:
```c++
#include<iostream>
using namespace std;
void showflags(long f) {
long i = 0x8000;
for (; i; i = i >> 1) {
if (i & f)
cout << "1";
else
cout << "0";
}
cout << endl;
}
int main() {
showflags(cout.flags()); // 输出流对象的标志位,使用 showflags 函数实现
cout << "x_width=" << cout.width() << endl; // 输出宽度
cout << "x_fill=" << cout.fill() << endl; // 输出填充字符
cout << "x_precision=" << cout.precision() << endl; // 输出精度
cout << 123 << " " << 123.45678 << "\n"; // 输出数值 123 和 123.45678
cout << "-------------" << endl;
cout << "* * * x_width=10,x_fill= ,x_precision=4 * * *" << endl<<endl<<endl<<endl;
cout.width(10); // 设置宽度为 10
cout.precision(4); // 设置精度为 4
cout << 123 << " " << 123.45678 << " " << 234.567 << endl; // 输出数值 123、123.45678 和 234.567
cout << "x_width=" << cout.width() << endl; // 输出宽度
cout << "x_fill=" << cout.fill() << endl; // 输出填充字符
cout << "x_precision=" << cout.precision() << endl;
cout << "-------------" << endl;
cout << "* * * x_width=10,x_fill=&,x_precision=4 * * *" << endl;
cout.fill('&'); // 设置填充字符为 &
cout.width(10); // 设置宽度为 10
cout << 123 << " " << 123.45678 << endl; // 输出数值 123 和 123.45678
cout.setf(ios::left); // 设置左对齐
cout.width(10); // 设置宽度为 10
cout << 123 << " " << 123.45678 << endl; // 输出数值 123 和 123.45678
cout << "x_width=" << cout.width() << endl; // 输出宽度
cout << "x_fill=" << cout.fill() << endl; // 输出填充字符
cout << "x_precision=" << cout.precision() << endl<<endl<<endl;
showflags(cout.flags()); // 输出流对象的标志位
cout.setf(ios::right | ios::unitbuf); // 设置右对齐和刷新缓冲区
cout << endl << endl << endl;
showflags(cout.flags()); // 输出流对象的标志位
}
```
阅读全文
相关推荐
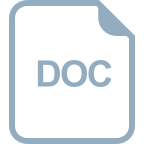
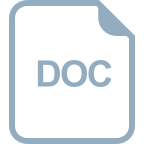







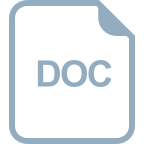
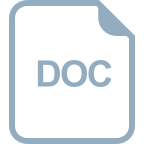
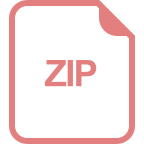
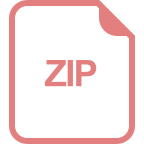
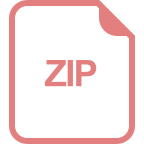
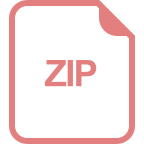
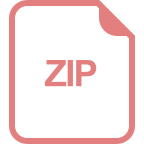
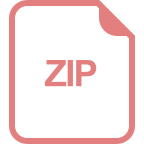