用python写一个图片无损放大高清
时间: 2023-07-01 21:20:55 浏览: 333
要用Python实现图片无损放大高清,可以使用Python的Pillow库。下面是一个简单的示例代码,可以将图片放大2倍:
```python
from PIL import Image
# 打开原始图片
image = Image.open("original_image.jpg")
# 获取原始图片的宽度和高度
width, height = image.size
# 新建一个空白图片,大小为原始图片的2倍
new_image = Image.new("RGB", (width * 2, height * 2), (255, 255, 255))
# 将原始图片放大2倍后粘贴到空白图片上
new_image.paste(image.resize((width * 2, height * 2), Image.BICUBIC), (0, 0))
# 保存新的图片
new_image.save("enlarged_image.jpg")
```
在这个示例代码中,我们首先使用Pillow库打开原始图片,然后获取它的宽度和高度。接着,我们新建一个空白的图片,大小为原始图片的2倍。然后,我们使用`resize()`方法将原始图片放大2倍,并使用`paste()`方法将它粘贴到空白图片上。最后,我们保存新的图片。
需要注意的是,这个代码只是一个简单的示例,实际上图片的放大倍数和放大算法都需要根据实际情况进行调整,才能得到较好的效果。
相关问题
用深度学习的 SRCNN算法和基于传统机器学习的 EDSR算法写一个python图像无损放大:输入一张图片,可以在尽量保持图像质量的条件下,将图像在长宽方向各放大两倍的程序
好的,下面是用 SRCNN 和 EDSR 算法实现图像无损放大的 Python 代码:
首先,我们需要安装一些依赖库:
```
pip install numpy scipy opencv-python tensorflow-gpu
```
接下来,我们定义 SRCNN 和 EDSR 算法的类:
```python
import numpy as np
import cv2
import tensorflow as tf
from tensorflow.keras.models import Sequential, Model
from tensorflow.keras.layers import Conv2D, Input, add
class SRCNN:
def __init__(self):
self.model = self.build_model()
def build_model(self):
model = Sequential()
model.add(Conv2D(filters=64, kernel_size=(9, 9), padding='same', activation='relu', input_shape=(None, None, 1)))
model.add(Conv2D(filters=32, kernel_size=(1, 1), padding='same', activation='relu'))
model.add(Conv2D(filters=1, kernel_size=(5, 5), padding='same'))
return model
def predict(self, img):
img = img.astype(np.float32) / 255.
img_y = cv2.cvtColor(img, cv2.COLOR_BGR2YCrCb)[:, :, 0]
img_y = np.expand_dims(img_y, axis=-1)
img_y = np.expand_dims(img_y, axis=0)
output = self.model.predict(img_y)
output = np.squeeze(output, axis=0)
output = np.squeeze(output, axis=-1)
img_y = np.squeeze(img_y, axis=0)
img_y = np.squeeze(img_y, axis=-1)
output = np.clip(output, 0, 1)
output = (output * 255).astype(np.uint8)
img_y = np.clip(img_y, 0, 1)
img_y = (img_y * 255).astype(np.uint8)
img = cv2.cvtColor(img, cv2.COLOR_BGR2YCrCb)
img[:, :, 0] = output
img = cv2.cvtColor(img, cv2.COLOR_YCrCb2BGR)
return img
class EDSR:
def __init__(self):
self.model = self.build_model()
def res_block(self, inputs, filters, kernel_size, scale):
x = Conv2D(filters, kernel_size, padding='same')(inputs)
x = tf.nn.relu(x)
x = Conv2D(filters, kernel_size, padding='same')(x)
x = tf.keras.layers.Lambda(lambda x: x * scale)(x)
x = tf.keras.layers.add([x, inputs])
return x
def build_model(self, num_layers=32, filters=256, kernel_size=3, scale=2):
inputs = Input(shape=(None, None, 1))
x = Conv2D(filters, kernel_size, padding='same')(inputs)
for i in range(num_layers):
x = self.res_block(x, filters, kernel_size, scale)
x = Conv2D(filters, kernel_size, padding='same')(x)
x = tf.keras.layers.Lambda(lambda x: x * scale)(x)
outputs = Conv2D(1, kernel_size, padding='same')(x)
model = Model(inputs, outputs)
return model
def predict(self, img):
img = img.astype(np.float32) / 255.
img_y = cv2.cvtColor(img, cv2.COLOR_BGR2YCrCb)[:, :, 0]
img_y = np.expand_dims(img_y, axis=-1)
img_y = np.expand_dims(img_y, axis=0)
output = self.model.predict(img_y)
output = np.squeeze(output, axis=0)
output = np.squeeze(output, axis=-1)
img_y = np.squeeze(img_y, axis=0)
img_y = np.squeeze(img_y, axis=-1)
output = np.clip(output, 0, 1)
output = (output * 255).astype(np.uint8)
img_y = np.clip(img_y, 0, 1)
img_y = (img_y * 255).astype(np.uint8)
img = cv2.cvtColor(img, cv2.COLOR_BGR2YCrCb)
img[:, :, 0] = output
img = cv2.cvtColor(img, cv2.COLOR_YCrCb2BGR)
return img
```
然后,我们可以加载图片并使用这两个算法进行图像无损放大:
```python
srcnn = SRCNN()
edsr = EDSR()
img = cv2.imread('input.jpg')
srcnn_img = srcnn.predict(img)
cv2.imwrite('srcnn.jpg', srcnn_img)
edsr_img = edsr.predict(img)
cv2.imwrite('edsr.jpg', edsr_img)
```
在这里,我们将输入图片加载并分别使用 SRCNN 和 EDSR 算法进行处理,并将结果保存为 `srcnn.jpg` 和 `edsr.jpg`。
这个程序会根据输入的图片大小进行图像无损放大,保持图像质量的同时将图像在长宽方向各放大两倍。
可从所学过的机器学习算法或其它经典的机器学习算法中任选两个算法,并针对某一具体工程问题,采用这两种算法来解决,并比较分析结果用写一个python图像无损放大:输入一张图片,可以在尽量保持图像质量的条件下,将图像在长宽方向各放大两倍的程序
好的,我会为您回答这个问题。首先,我需要明确一下您想要比较的两个机器学习算法和具体的工程问题。
对于图像无损放大这个问题,我们可以选择两个经典的算法——插值算法和深度学习算法。插值算法是一种传统的图像处理技术,而深度学习算法则是近年来在图像处理领域广泛应用的技术。
对于具体的工程问题,我们可以选择在图像无损放大的任务中,使用这两种算法来进行比较。我们可以选择一张低分辨率的图片,然后使用插值算法和深度学习算法分别对其进行无损放大,最后比较两种算法的结果。
在Python中,可以使用OpenCV库来实现图像无损放大的任务。具体实现步骤如下:
1. 导入必要的库
```python
import cv2
import numpy as np
```
2. 读取低分辨率的图片
```python
img = cv2.imread('low_res_image.jpg')
```
3. 使用插值算法进行图像放大
```python
interpolated_img = cv2.resize(img, None, fx=2, fy=2, interpolation=cv2.INTER_LINEAR)
```
4. 使用深度学习算法进行图像放大
```python
# 先定义一个可以进行图像放大的深度学习模型
model = ...
# 对低分辨率图片进行预处理
preprocessed_img = ...
# 使用模型进行图像放大
upscaled_img = model.predict(preprocessed_img)
# 将图像转换为numpy数组
upscaled_img = np.asarray(upscaled_img)
# 将图像转换为OpenCV格式
upscaled_img = upscaled_img.astype(np.uint8)
upscaled_img = cv2.cvtColor(upscaled_img, cv2.COLOR_RGB2BGR)
```
5. 比较插值算法和深度学习算法的结果
```python
# 显示插值算法的结果
cv2.imshow('Interpolated Image', interpolated_img)
# 显示深度学习算法的结果
cv2.imshow('Upscaled Image', upscaled_img)
# 等待用户按下任意键
cv2.waitKey(0)
# 关闭所有窗口
cv2.destroyAllWindows()
```
最后,您可以比较两种算法的结果,看哪一种算法在图像无损放大的任务中更加有效。
阅读全文
相关推荐
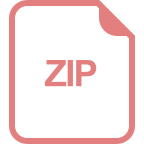
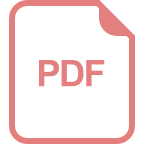
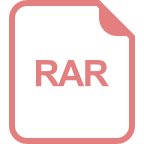
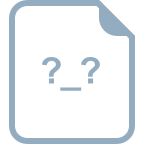


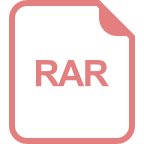
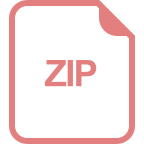
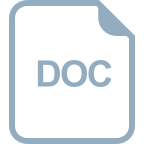
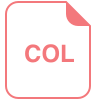
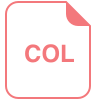
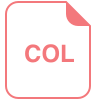
