用python写一个读取摄像头并存图的代码
时间: 2024-05-15 11:17:06 浏览: 92
以下是一个简单的Python代码,用于读取摄像头并将图像保存在本地文件中:
```python
import cv2
# 打开相机
cap = cv2.VideoCapture(0)
# 设置图片尺寸
cap.set(cv2.CAP_PROP_FRAME_WIDTH, 640)
cap.set(cv2.CAP_PROP_FRAME_HEIGHT, 480)
# 循环读取相机数据
while True:
# 读取一帧数据
ret, frame = cap.read()
# 显示图像
cv2.imshow('camera', frame)
# 等待按下ESC键退出程序
if cv2.waitKey(1) == 27:
break
# 按下空格键保存图像
if cv2.waitKey(1) == ord(' '):
filename = 'camera_image.jpg'
cv2.imwrite(filename, frame)
print('Image saved as', filename)
# 释放资源
cap.release()
cv2.destroyAllWindows()
```
该程序使用OpenCV库打开相机并设置图像尺寸,然后循环读取相机数据并显示图像。当按下空格键时,程序将当前图像保存为本地文件,并在控制台中输出文件名。当按下ESC键时,程序退出并释放相机资源。
相关问题
编写python 代码,用摄像头抓取实验者自己的彩色图像,转换为灰度图像,然后在屏幕的8个区域中分别显示该图像的256、128、64、32、16、8、4、2色图像,并存成磁盘文件
```python
import cv2
# 打开摄像头
cap = cv2.VideoCapture(0)
# 读取图像
ret, frame = cap.read()
# 转换为灰度图像
gray = cv2.cvtColor(frame, cv2.COLOR_BGR2GRAY)
# 显示灰度图像
cv2.imshow('gray', gray)
# 分别显示8个区域中的不同颜色图像
for i in range(8):
color = 2**(i+1)
img = (gray // color) * color
cv2.imshow(f'{color}-color', img)
# 保存图像到磁盘文件
filename = f'{color}-color.jpg'
cv2.imwrite(filename, img)
# 等待按下任意键退出程序
cv2.waitKey(0)
# 释放摄像头并关闭窗口
cap.release()
cv2.destroyAllWindows()
```
注意:在运行上述代码时,需要保证电脑上有摄像头设备,并且已经安装好了OpenCV库。如果没有安装,可以通过以下命令在终端中安装:
```
pip install opencv-python
```
用摄像头抓取实验者自己的彩色图像,转换为灰度图像,然后在一个窗口平分的8个区域中分别显示该图像的256、128、64、32、16、8、4、2色图像,并存成磁盘文件,用python给出代码并给出注释
```python
import cv2
# 读取彩色图像
img = cv2.imread("test.jpg")
# 转换为灰度图像
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
# 定义颜色数量列表
color_nums = [256, 128, 64, 32, 16, 8, 4, 2]
# 循环生成不同颜色数量的图像并显示并存储
for i, num in enumerate(color_nums):
# 利用cv2的量化函数将灰度图像转换为指定颜色数量的图像
img_quantized = cv2.quantize(gray, colors=num, kmeansCenters=None)
# 将量化结果转换为图像格式
img_quantized = cv2.convertScaleAbs(img_quantized[0])
# 显示图像
cv2.imshow(f"{num} colors", img_quantized)
# 存储图像
cv2.imwrite(f"{num}_colors.jpg", img_quantized)
# 每个图像之间留有一定的间隔
cv2.moveWindow(f"{num} colors", (i % 4) * 250, (i // 4) * 300)
# 等待键盘输入
cv2.waitKey(0)
# 关闭所有窗口
cv2.destroyAllWindows()
```
注释见代码。
阅读全文
相关推荐
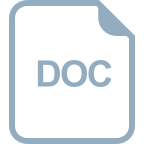
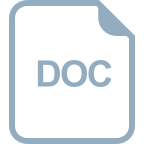
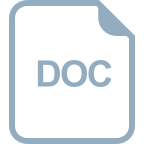
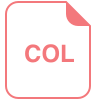
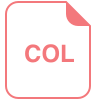

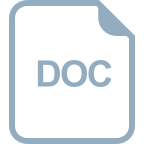
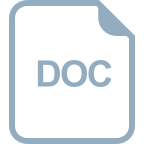
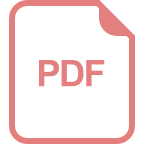
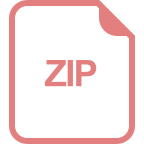
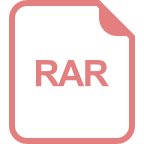
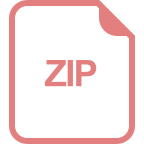
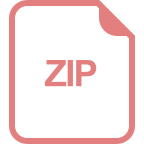
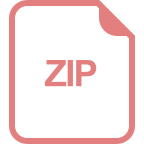
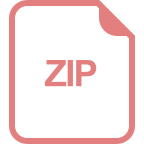
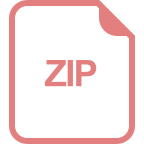
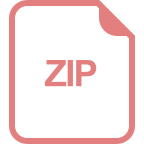