请用C语言写下面的代码,要求水果店有三种水果,价格为每公斤苹果9.8元,每公斤梨4.2元,每公斤香蕉2.3元,收银员输入每种水果购买重量,输出应付总金额
时间: 2023-03-24 15:05:07 浏览: 89
#include <stdio.h>
int main() {
float apple_price = 9.8;
float pear_price = 4.2;
float banana_price = 2.3;
float apple_weight, pear_weight, banana_weight;
float total_price;
printf("请输入苹果购买重量(kg):");
scanf("%f", &apple_weight);
printf("请输入梨购买重量(kg):");
scanf("%f", &pear_weight);
printf("请输入香蕉购买重量(kg):");
scanf("%f", &banana_weight);
total_price = apple_price * apple_weight + pear_price * pear_weight + banana_price * banana_weight;
printf("应付总金额为:%.2f元\n", total_price);
return ;
}
相关问题
请用C语言写下面的代码,要求如下输入一个正整数判断是否为素数
很高兴为您服务!以下是用C语言写判断素数的代码:
```
#include <stdio.h>
int main()
{
int num, i, flag = 0;
printf("请输入一个正整数:");
scanf("%d", &num);
for(i=2; i<=num/2; i++)
{
if(num%i == 0)
{
flag = 1;
break;
}
}
if(flag == 0 && num > 1)
printf("%d是素数。\n", num);
else
printf("%d不是素数。\n", num);
return 0;
}
```
输入一个正整数,程序会判断它是否为素数,最后输出判断结果。如果输入的是素数,则输出“X是素数。”,其中X为输入的正整数;如果输入的不是素数,则输出“X不是素数。”。希望可以满足您的要求。
用c语言写,出租车三公里内八元,之后每公里1.6元,计算费用
好的,根据您的要求,我为您编写了以下的C语言代码,可以计算出租车的费用:
```c
#include <stdio.h>
int main()
{
float distance, cost;
printf("请输入行驶的公里数:");
scanf("%f", &distance);
if (distance <= 3) {
cost = 8;
} else {
cost = 8 + (distance - 3) * 1.6;
}
printf("您需要支付的费用为:%.2f元\n", cost);
return 0;
}
```
在代码中,我们首先定义了两个变量 `distance` 和 `cost`,分别用于存储行驶的公里数和需要支付的费用。然后,通过 `scanf` 函数提示用户输入行驶的公里数,并将其存储到 `distance` 变量中。接下来,使用 `if-else` 语句来判断行驶的公里数是否小于等于 3 公里,如果是,则费用为 8 元,否则费用为 8 元加上超出 3 公里的部分乘以每公里 1.6 元的费用。最后,通过 `printf` 函数输出需要支付的费用。
希望这个代码可以帮助您解决问题,如果您有任何其他问题,请随时提出。
相关推荐
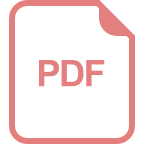












