写一份卷积收缩自动编码器的pytorch版本代码
时间: 2023-09-27 21:06:54 浏览: 111
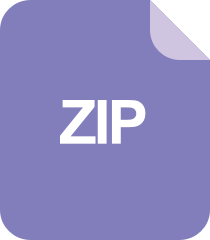
基于PyTorch的图自动编码器示例.zip
好的,以下是使用 PyTorch 实现卷积收缩自动编码器的代码:
```python
import torch
import torch.nn as nn
# 定义卷积收缩自动编码器的类
class ConvolutionalContractiveAutoencoder(nn.Module):
def __init__(self, input_shape, code_size, alpha=1e-4):
super(ConvolutionalContractiveAutoencoder, self).__init__()
# 定义模型的输入输出和编码器参数
self.input_shape = input_shape
self.code_size = code_size
self.alpha = alpha
# 定义编码器
self.encoder = nn.Sequential(
nn.Conv2d(input_shape[2], 32, kernel_size=(3, 3), stride=(2, 2), padding=1),
nn.ReLU(),
nn.Conv2d(32, 64, kernel_size=(3, 3), stride=(2, 2), padding=1),
nn.ReLU(),
nn.Flatten(),
nn.Linear(64*(input_shape[0]//4)*(input_shape[1]//4), code_size),
nn.ReLU()
)
# 定义解码器
self.decoder = nn.Sequential(
nn.Linear(code_size, 64*(input_shape[0]//4)*(input_shape[1]//4)),
nn.ReLU(),
nn.Unflatten(1, (64, input_shape[0]//4, input_shape[1]//4)),
nn.ConvTranspose2d(64, 32, kernel_size=(3, 3), stride=(2, 2), padding=1, output_padding=1),
nn.ReLU(),
nn.ConvTranspose2d(32, input_shape[2], kernel_size=(3, 3), stride=(2, 2), padding=1, output_padding=1)
)
def forward(self, x):
# 计算编码器输出和解码器输出
encoded = self.encoder(x)
decoded = self.decoder(encoded)
# 计算损失函数
loss = nn.MSELoss()(x, decoded)
jacobian = torch.autograd.functional.jacobian(encoded, x).view(-1, self.code_size, self.input_shape[0]*self.input_shape[1])
jacobian = torch.bmm(jacobian, jacobian.transpose(1, 2)).view(-1, self.code_size*self.code_size)
loss += self.alpha * torch.mean(torch.sum(jacobian**2, dim=1))
return decoded, loss
```
这个代码同样实现了一个基于卷积神经网络的收缩自动编码器。我们使用了 PyTorch 中的 `nn.Sequential` 模块来定义卷积和全连接层,使用了 `nn.MSELoss` 来计算损失函数,使用了 `torch.autograd.functional.jacobian` 来计算雅可比矩阵,并使用 PyTorch 中的运算符 `view`、`transpose`、`bmm` 和 `mean` 等来对张量进行操作。
阅读全文
相关推荐
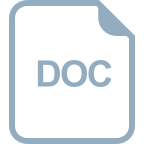
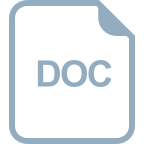
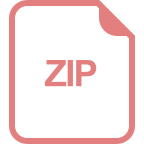
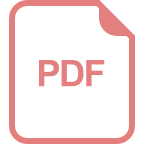
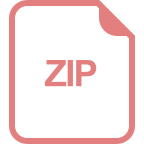
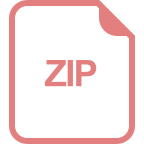
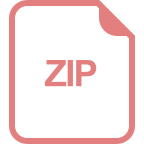
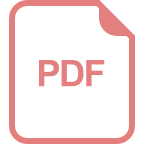
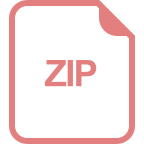
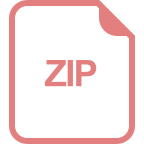
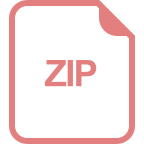
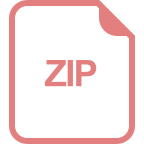
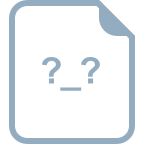
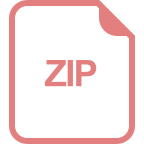
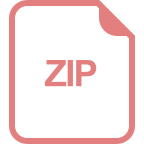
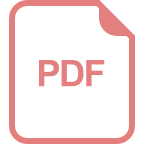
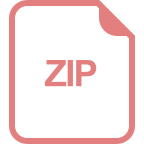